dannyelo
CCConvex Community
•Created by dannyelo on 10/3/2024 in #support-community
Search Index by Two or More Fields
Hello, I'm trying to retrive a query using searchIndex.
I need it to query documents by two or more fields.
This is what I tried so far, but is only retriving results for legalName.
19 replies
CCConvex Community
•Created by dannyelo on 10/1/2024 in #support-community
Type error: implicitly has type 'any' because it does not have a type annotation
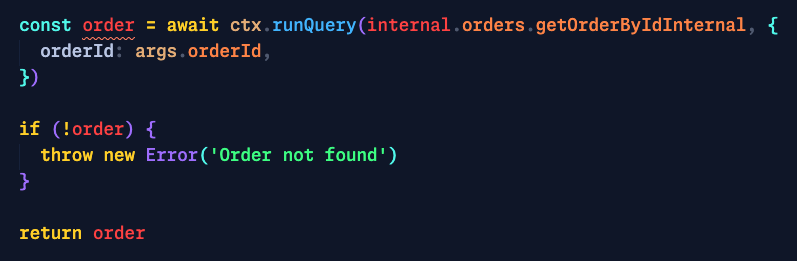
44 replies
CCConvex Community
•Created by dannyelo on 9/26/2024 in #support-community
runAfter is not throwing an error on the client
Hello, can't understand why error is not throwing to the client.
This is the pattern I'm trying to implement.
I can see the Error 'Called getCurrentUser without authentication present' logged in convex, but I can't get it in the front end. I'm using Next.js.
convex/customers.ts
convex/customers_usenode.ts
convex/facturapi_usenode.ts
121 replies
CCConvex Community
•Created by dannyelo on 9/23/2024 in #support-community
Optimizing Function Calls by Avoiding Redundant Abstractions
The problem I’m facing is that I’m using an extra layer of abstraction in my code that doesn’t seem necessary. I have a function called
getCurrentUserInternal
, which is basically just a wrapper around another function called getCurrentUser
. I’m using this wrapper to access user information inside another function, getFacturapiLiveInstance
.
The problem is that getCurrentUser accepts QueryCtx, and in the action I only have access to ActionCtx.
Is there a better way?
convex/users.ts
convex/facturapi_usenode.ts
6 replies
CCConvex Community
•Created by dannyelo on 9/18/2024 in #support-community
Query Convex data from Next.js route.ts endpoint
Can I query data from Convex db in next.js
route.ts
endpoint?
app/api/endpoint/route.ts
4 replies
CCConvex Community
•Created by dannyelo on 9/17/2024 in #support-community
Access the action error message when fail
Hello,
When I call an action that make a mutation to an external API, and the request fails, I get a Convex Error string, but I need to access the API response error message.
How can I access only the error string? In this case:
El certificado no es un CSD. Asegúrate de no estar enviando una FIEL.
5 replies
CCConvex Community
•Created by dannyelo on 9/16/2024 in #support-community
External API fetch advise
Hello, I'm using Convex folder for all my data-access layer now. I need to fetch some data from other APIs and they don't interact with Convex. I'm using Next.js. Is there any downside if I use an action in a 'use node' file for fetching data, or is better to use a next.js route instead?
13 replies
CCConvex Community
•Created by dannyelo on 9/13/2024 in #support-community
Call internalAction in 'use node' environment, from a mutation
Hello, how can I call internalAction from a mutation, In the mutation ctx I don't have any option to do it. Is this possible?
3 replies
CCConvex Community
•Created by dannyelo on 9/4/2024 in #support-community
Help using Convex Action
I'm trying to use an action because I'm calling external SDK and cryptr npm package.
But I got an error, I can't find what is wrong.
convex/facturapi.ts
convex/invoices.ts
Error
8 replies
CCConvex Community
•Created by dannyelo on 9/3/2024 in #support-community
Encrypt organizations secrets
I'm using an external API, where I'm going to create a 1:1 relationship between the organizations in my database and the organizations created in the API. For each request in the external API, I need a secret key per organization for making requests.
I need to store in my database the secret key for each organization, but I need to encrypt it.
I was thinking of using Cryptr (npm), but reading the Convex documentation, I see that not all npm packages are accepted by convex.
Any recommendations would be greatly appreciated.
5 replies
CCConvex Community
•Created by dannyelo on 8/29/2024 in #support-community
[ERROR] Could not resolve "node:async_hooks"
Hello,
I have this error when trying to run
npx convex dev
Any idea?
4 replies
CCConvex Community
•Created by dannyelo on 8/27/2024 in #support-community
Cleanest way to handle optional filters
Hello,
I need to filter orders table by
status
/orders
route should get all orders
/orders?status=pending
should get orders with the status of 'pending'
What should I pass to ignore the filter?
I tried this and is not working.
11 replies
CCConvex Community
•Created by dannyelo on 8/24/2024 in #support-community
Extracted function types
Hello! I extracted a function to map a query. But I can't find the correct types.
I'm looking for the type
order
, orderLine
, ctx
and q
.
order
and orderLine
are raw records in the database.
3 replies
CCConvex Community
•Created by dannyelo on 8/20/2024 in #support-community
Zod and Convex type error
Hello,
I'm using Zod to validate my forms.
When I pass the form data to a mutation function, I got a type error.
The function expect some convex ids and the zod schema is a simple string.
What is the fix here?
Zod Schema
Expecting
Error
4 replies
CCConvex Community
•Created by dannyelo on 8/16/2024 in #support-community
Best way to handle this ts error using useQuery()
Hello, I want to know what advise can you give me to handle this situation when using useQuery.
Example code
TS Error in (organizationId):
3 replies
CCConvex Community
•Created by dannyelo on 8/12/2024 in #support-community
Insert a record, then another record with the id of the first one in the same transaction
Hello,
I have some tables
orders
and order_products
This is the schema:
I'm trying to construct a create function but I'm not sure how to get the orderId first to assign it to all the order_products
Is this the correct approach?
Thanks!11 replies
CCConvex Community
•Created by dannyelo on 8/9/2024 in #support-community
Query organizations that belong to one user
Hello,
I'm trying to query multiple organizations a user is assigned.
Here I share my code.
Is this the best way to make this types of queries?
Thanks!
schema.ts
organizations.ts
3 replies
CCConvex Community
•Created by dannyelo on 7/29/2024 in #support-community
.jpg images are uploading with 0 KB while .webp are OK
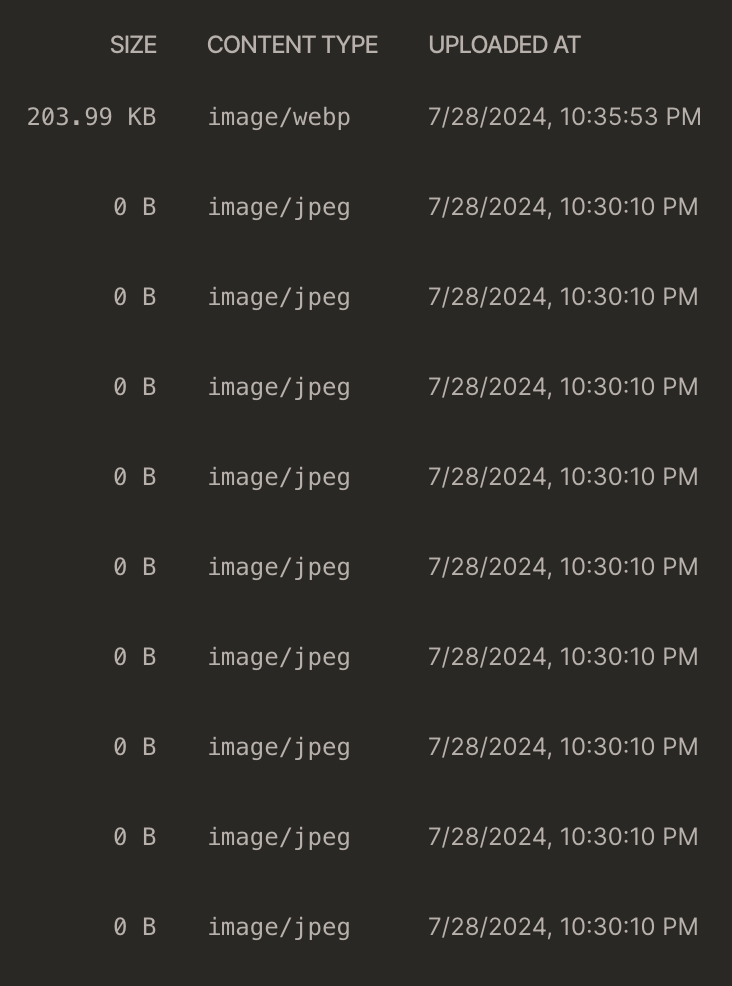
1 replies
CCConvex Community
•Created by dannyelo on 7/29/2024 in #support-community
Need help using the uploadstuff api
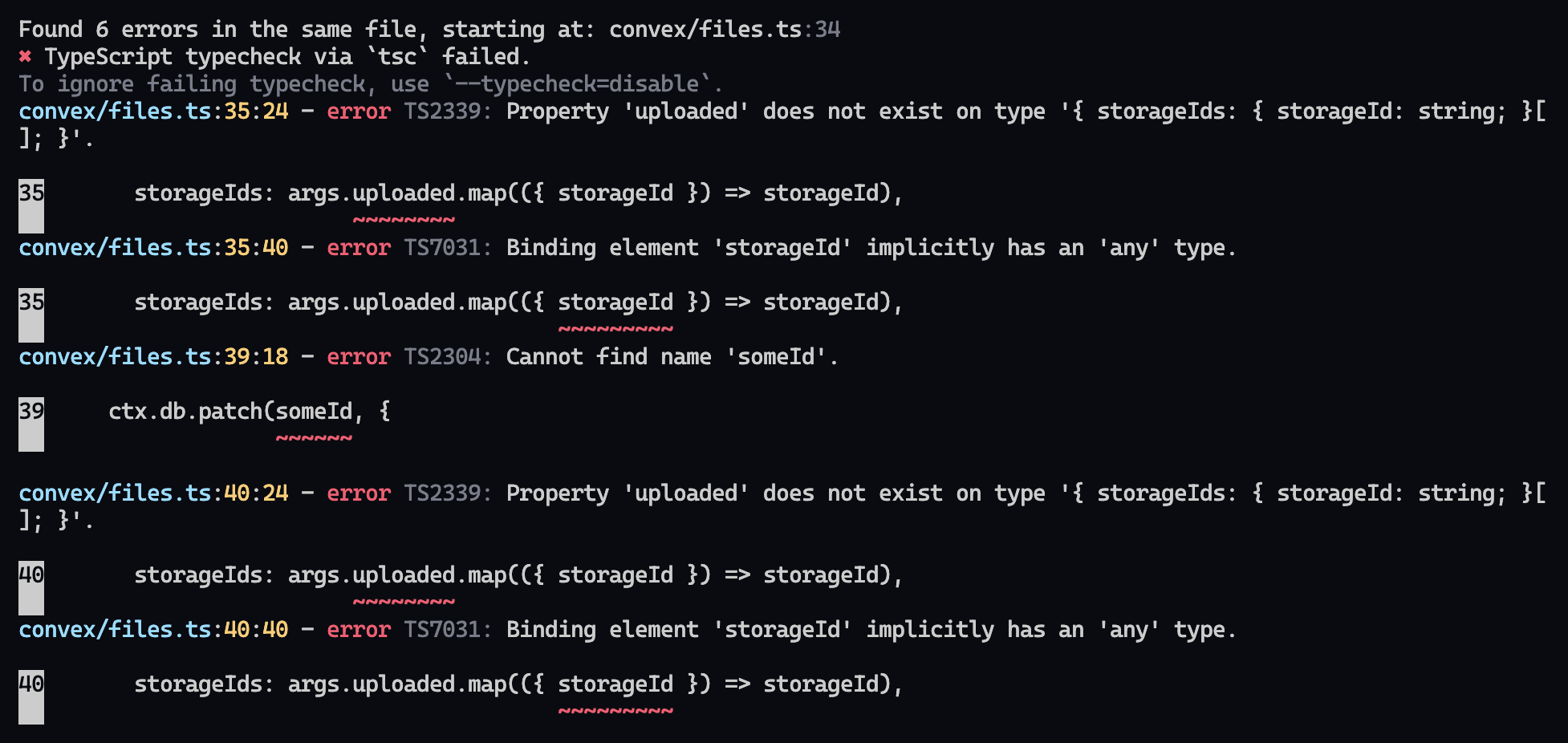
43 replies
CCConvex Community
•Created by dannyelo on 7/27/2024 in #support-community
v.string().isOptional property not working
Hello,
I saw available the isOptional property in the v.string()
But I have an error after I use it in the property name.
Any suggestion?
5 replies