Cleanest way to handle optional filters
Hello,
I need to filter orders table by
status
/orders
route should get all orders
/orders?status=pending
should get orders with the status of 'pending'
What should I pass to ignore the filter?
I tried this and is not working.
9 Replies
This code is working but is not optimal
You could try storing your query in a variable, and adding filters onto it:
Also, have you read about Convex indexes yet? They're a great way to make your queries more performant, and you can do conditional chaining similar to the example I gave above (but with slightly differenct syntax). docs here: https://docs.convex.dev/database/indexes/#querying-documents-using-indexes
Indexes | Convex Developer Hub
Indexes are a data structure that allow you to speed up your
+1 to what @ari said: indexes and dynamic filter construction are great. The quickest answer to your initial question is instead of
return null
you can return true
to disable a filterThank you @ari and @lee!
hey @ari @lee i have a question that extends to this one, what if i have multiple parameter to filter from (other than status).
i know that there's combined operator like
q.and
. but in this case, all the parameters is optional. am i expected to catch all the possibilities
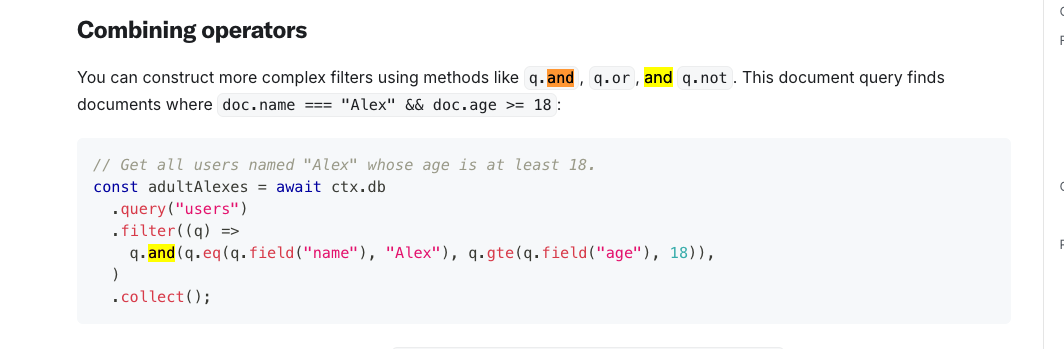
thanks for your sugggestion @M Zeeshan. whats the difference between thisand the combined operator?
This way you can filter only when filter1, filter2 or filter3 is defined. e.g. filter 1 is defined but filter2 and filter3 both are undefined they will not participate in filter.
https://discord.com/channels/1019350475847499849/1284787456373297152/1284792305009688576