Insert a record, then another record with the id of the first one in the same transaction
Hello,
I have some tables
orders
and order_products
This is the schema:
I'm trying to construct a create function but I'm not sure how to get the orderId first to assign it to all the order_products
Is this the correct approach?
Thanks!8 Replies
yes that looks right
(you should await your promises though)
Thanks @lee! I'm awaiting them, aren't I?
args.products.forEach(async (product) => {
this will create a lot of promises that don't get awaitedHow can I await them?
Like this?
that's close
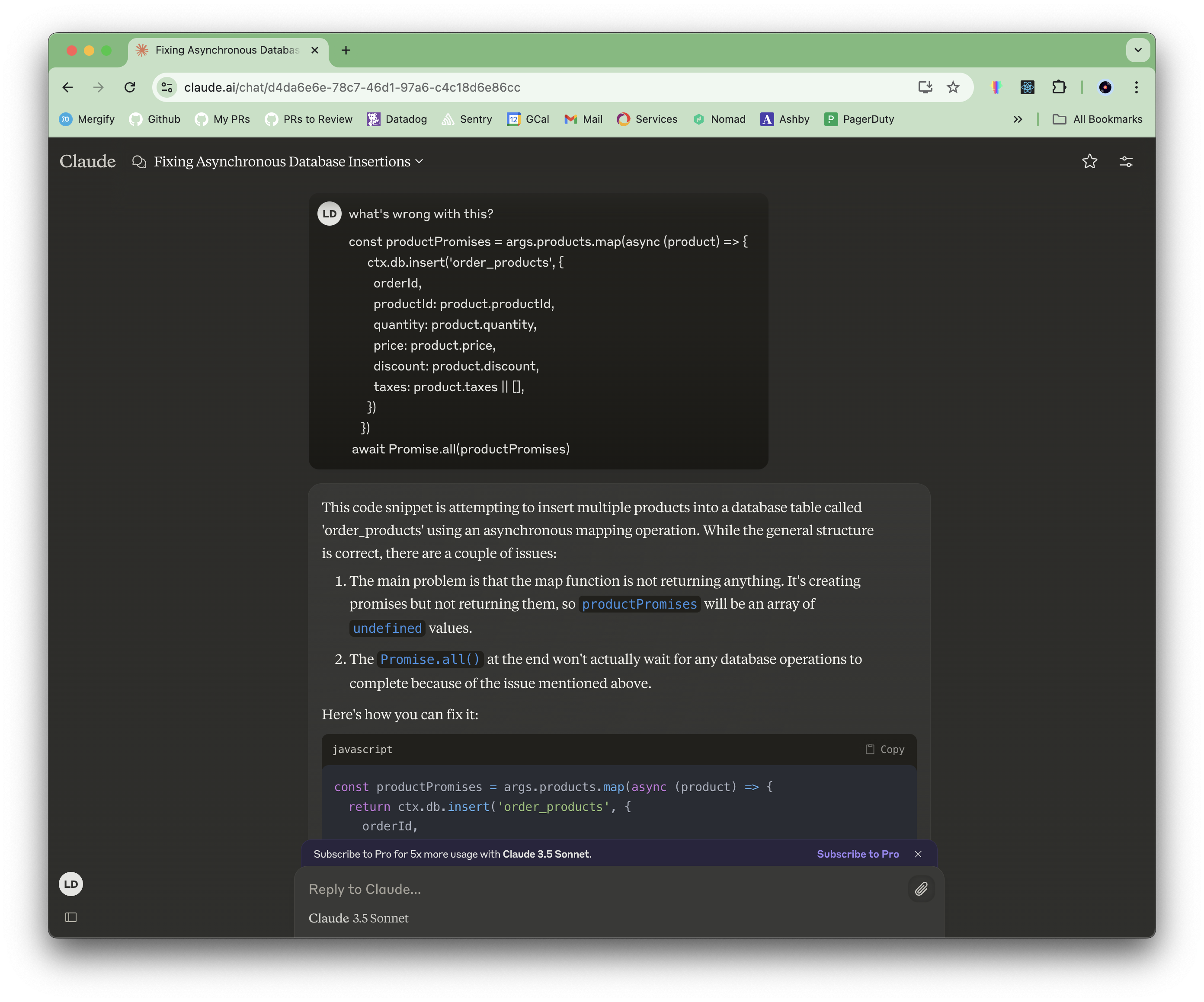
Oh I forgot to return each insertion
yep that's good 🙂
Thank you @lee!!