sonandmjy
CCConvex Community
•Created by sonandmjy on 4/3/2025 in #support-community
Typescript inference help in monorepo
nevermind I think it was a typescript limitation, once I made the convex backend into a compiled package and exported the .d.ts files the type inference came back
2 replies
CCConvex Community
•Created by atadan on 4/3/2025 in #support-community
r2/actionRetrier cleanupExpiredRuns cron job stuck at loop
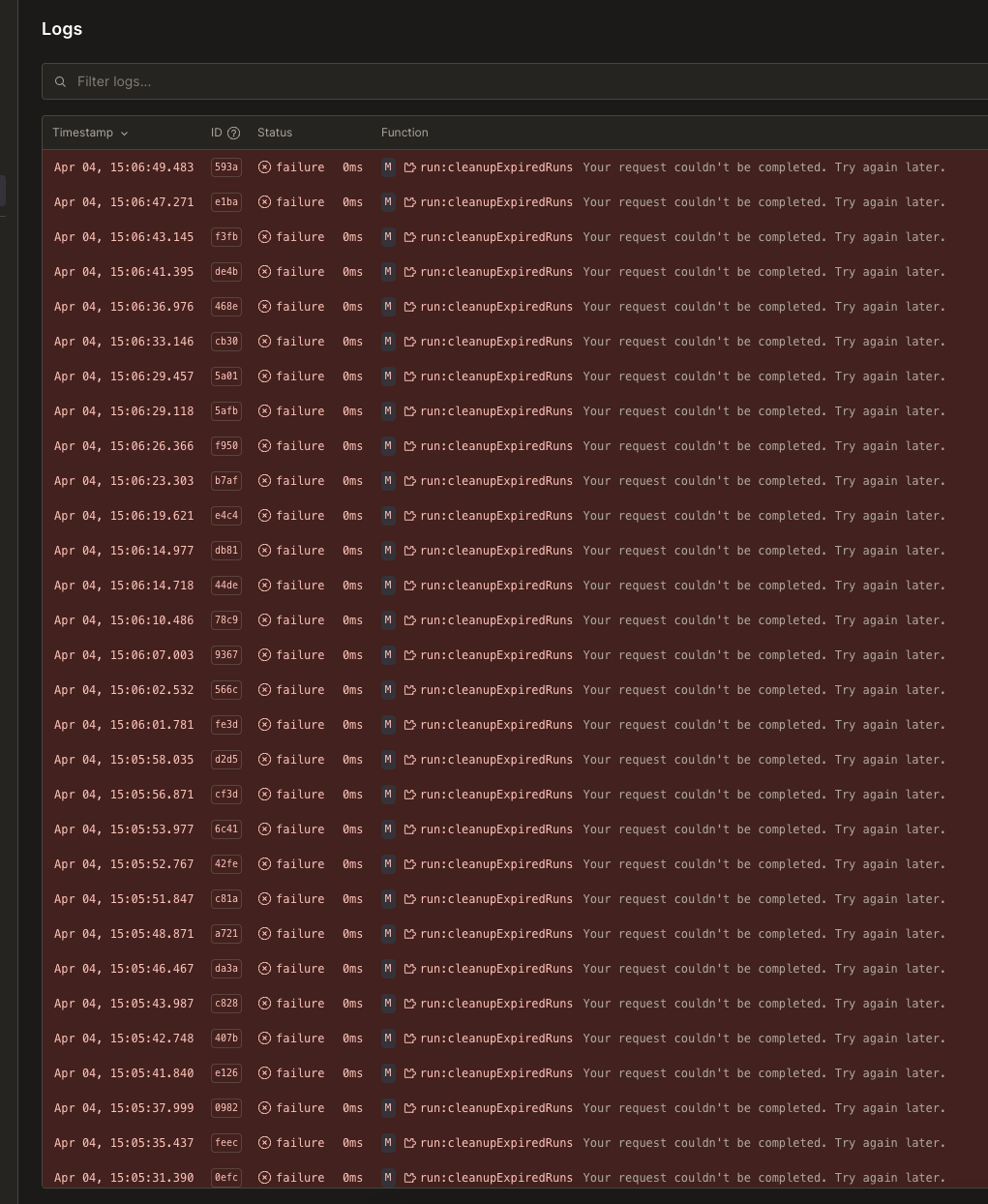
60 replies
CCConvex Community
•Created by Sara on 1/30/2025 in #support-community
while using pagination, I'm failing to sort the elements in the right order of creation
I'm did something similar before and it worked for me, mostly trusted convex to do the ordering for me but i did do some grouping in client side. This is my code if it helps
6 replies
CCConvex Community
•Created by too_easy on 2/7/2025 in #support-community
Could not load the "sharp" module using the linux-arm64 runtime
I’ve tried it with npm before and it works but i believe u have to use the node runtime and u have to externalise the module
node runtime
https://docs.convex.dev/functions/runtimes
externalise
https://docs.convex.dev/functions/bundling#external-packages
5 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
also idk if this is a good idea but I may also experiment with replacing the live updates for this particular use case using another service like liveblocks or sth even though convex has is realtime by default because seems kind of expensive if I am subscribing to every change to every message to keep my indexDB layer in sync 🤔
11 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
the apiService.getMessagesByDestinationAndChannelId is basically just wrapping the convex client (underneath it is calling the endpoint with getPage)
11 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
Hi Lee, I'm actually trying to do data replication using rx-db, the above is some semi-working code I am doing right now. So I am making a chat app. I successfully did it using the paginate fn already but I am now experimenting with a local first approach using rx-db. The idea I am running with is that i want to try to save the messages inside indexedDB when the user first load the conversation by paginating through them and saving them (that's what getPage is for) so when they next visit the page load can be instant. rx-db provides the help on handling conflicts, data replication, live updates etc. And I am orchestrating this all through xstate
11 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
11 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
thank you for the response!
11 replies
CCConvex Community
•Created by sonandmjy on 2/4/2025 in #support-community
filtering documents with getPage
oh I see, and it will only fetch results containing these two index keys if no endIndexKey is provided?
11 replies
CCConvex Community
•Created by sonandmjy on 1/21/2025 in #support-community
How would you handle third party subscriptions in convex
thanks for the clarification @Lee first time seeing a pattern like this so was wondering if it was something I was unaware about
5 replies
CCConvex Community
•Created by sonandmjy on 1/21/2025 in #support-community
How would you handle third party subscriptions in convex
ah that's what I thought, some of the things I want to do are heavy compute like video/image processing so i thought it might be better to do outside convex and send the result back
5 replies
CCConvex Community
•Created by sonandmjy on 12/25/2024 in #support-community
search index multiple chained filter fields behaviour not as expected
Not sure why but after a while it started working but I didn't change anything... not sure if convex has a cache or something that was causing it not to reflect the changes immediately
4 replies
CCConvex Community
•Created by amir on 12/25/2024 in #support-community
Query by array
I think u can add an index with walletAddress on the user table then loop over the walletAddresses array then fetch each user by index. If wallet address is unique in the table u can use the .unique() method as well.
4 replies
CCConvex Community
•Created by lexcodeit on 12/24/2024 in #support-community
Multiple Fields Names in Full Text Search
eg. if this is ur document in the DB
you would change the schema to become
and search the searchIndex instead of the firstName and lastName fields
5 replies
CCConvex Community
•Created by lexcodeit on 12/24/2024 in #support-community
Multiple Fields Names in Full Text Search
I think you can't rn, you need to create a new field and concat the two fields into a single field and seach that instead.
5 replies
CCConvex Community
•Created by sonandmjy on 12/21/2024 in #support-community
Hitting error "found wrong number of app bundles" in dev CLI
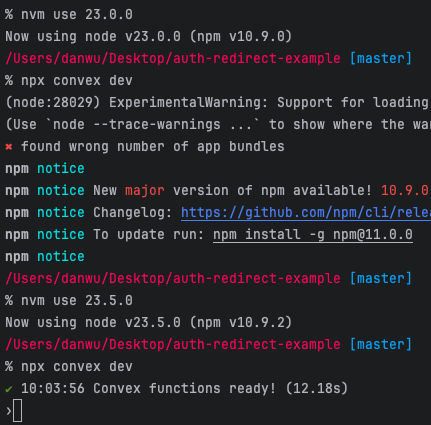
12 replies
CCConvex Community
•Created by sonandmjy on 12/21/2024 in #support-community
Hitting error "found wrong number of app bundles" in dev CLI
no worries, happy to share findings! @erquhart I did a quick test on 23.5.0 and it seems to start up fine, not sure what happened in 23.0.0 that caused it to break 🤔
12 replies
CCConvex Community
•Created by sonandmjy on 12/21/2024 in #support-community
Hitting error "found wrong number of app bundles" in dev CLI
FYI to anyone coming across this issue, it was because of node version. I was using node v23.0.0, it worked once i switched to v20.18.0 🤷♂️
12 replies
CCConvex Community
•Created by Eyal Alfasi on 10/22/2024 in #support-community
Authentication in Remix Loader with Convex Auth
alright, I've explained a bit more and linked this conversation to it. Hope to see it soon and happy to work on it too if you guys give me some pointers! Thank you
22 replies