Why is calling Convex queries/mutations directly an anti-pattern?
I'm currently working through refactoring my Convex queries and mutations to allow upgrading to 1.21, which throws a type error when you call Convex functions from other Convex functions.
The docs recommend relocating your functions into a
model/
directory, and then importing and calling those functions from your Convex mutations and queries.
I have a few questions:
1. Why is calling Convex functions from other functions an anti-pattern, specifically?
2. The examples in the docs take the logic from the original query/mutation and relocate it to a new function in a directory called model/
, and then import and call that function from the original mutation/query. I don't fully understand the rationale behind this, since now there are two separate files with similar/identical function names, and two places to test instead of one.
3. For maintainability, is the recommendation to relocate all query and mutation logic into a separate function within model/
? My first intuition was to only relocate the shared logic, but the docs seem to suggest otherwise?Best Practices | Convex Developer Hub
This is a list of best practices and common anti-patterns around using Convex.
23 Replies
Thanks for posting in <#1088161997662724167>.
Reminder: If you have a Convex Pro account, use the Convex Dashboard to file support tickets.
- Provide context: What are you trying to achieve, what is the end-user interaction, what are you seeing? (full error message, command output, etc.)
- Use search.convex.dev to search Docs, Stack, and Discord all at once.
- Additionally, you can post your questions in the Convex Community's <#1228095053885476985> channel to receive a response from AI.
- Avoid tagging staff unless specifically instructed.
Thank you!
Others will likely have more insight, but imo, it's about helping folks understand convex directory exports as api endpoints, rather than serverless functions that also happen to be endpoints. So the code in all convex directory files should be entirely focused on exposure of functionality, while the model directory is where all of the inner workings of your backend lives. The separation, and having a clear handle on what is and is not exposed to the public, is important. For a new/small project, this will feel like an annoyance, but at scale it's almost a requirement from a security and auditability standpoint.
i agree on @erquhart 's definitions and where the abstraction boundaries should be. in most frameworks I've used, once you expose an endpoint you probably won't call it internally; you'll refactor out a function. e.g. in flask https://stackoverflow.com/questions/43305658/proper-way-to-make-a-call-to-an-endpoint-from-the-api-using-flask
additionally, if you want more reasons why it's an antipattern, it's because when you wrap something in
query()
or mutation()
, we want that to mean it runs argument validation and runs as a transaction. And if you run such a function with useQuery
, useMutation
, ctx.runQuery
, ctx.runMutation
, ctx.scheduler.runAfter
, etc. all of that is true. But if you call it directly, it doesn't run argument validation or run in an isolated environment.helping folks understand convex directory exports as api endpoints, rather than serverless functions that also happen to be endpointsI knew this was a thing but it definitely wasn't the primary way I was conceptualizing
/convex
. That's helpful!
the code in all convex directory files should be entirely focused on exposure of functionality, while the model directory is where all of the inner workings of your backend livesTbh I'm not entirely sure where to draw that line between internal / external. It feels a little hazy, which is maybe part of what's leading to my confusion Probably a consequence of having spent most of my time as a frontend engineer and not a backend one. But slowly getting more familiar with how to build things for the backend, too!
If you need to access a given Convex function from a client, you'll need a public Convex function for it. If you need access from something other than a client, like a webhook or via scheduler from another function, but never from a client, then it should be internal. And if it's only run directly (not scheduled) by a Convex function and nothing more, then it should be a function in the model directory.
Probably a consequence of having spent most of my time as a frontend engineer and not a backend one.Precisely my perspective/experience as well
This is a handy rule, thank you!
Followup q: is the
/model
directory supposed to generate API routes?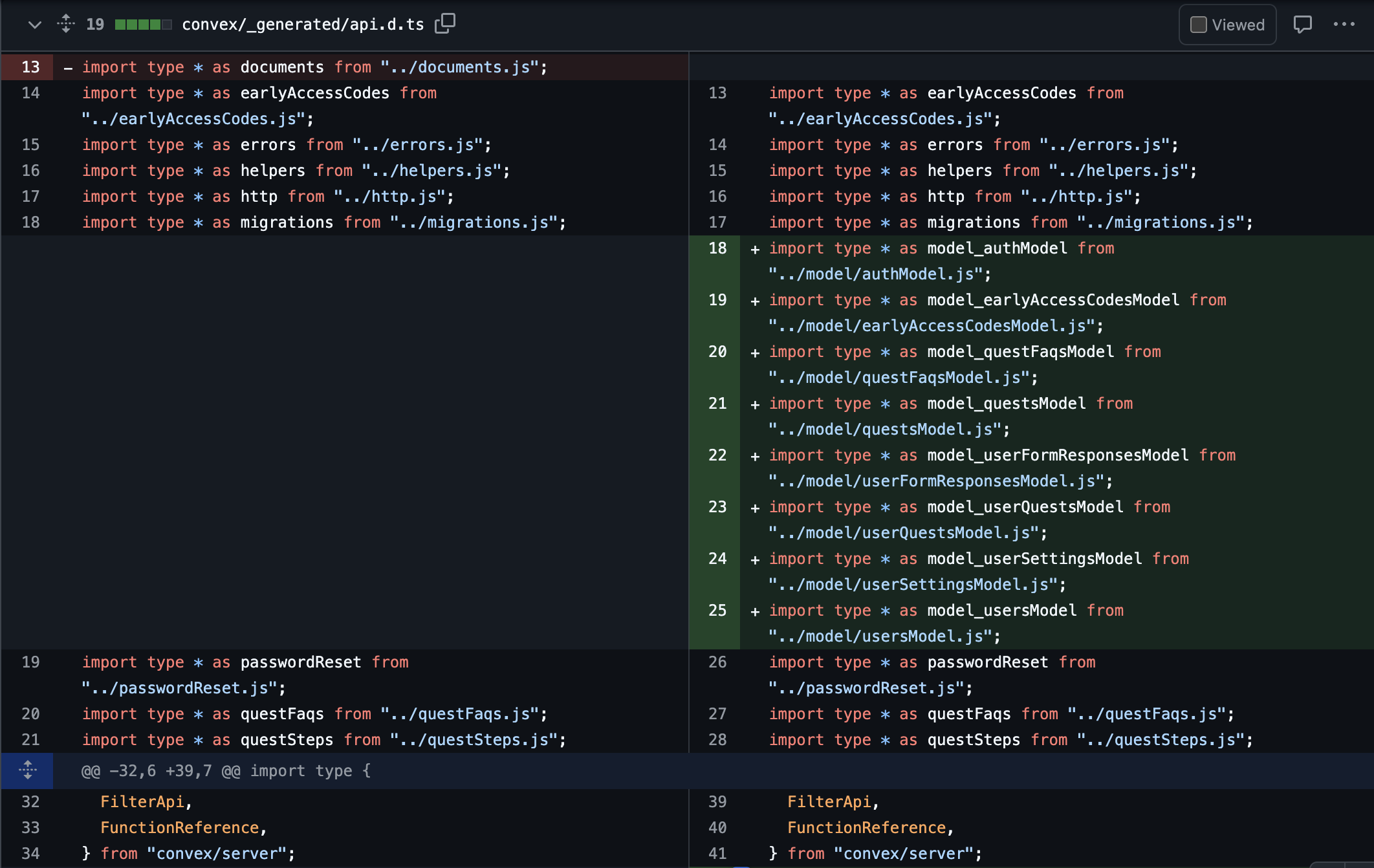
I relocated all the innards of my queries and mutations — anyone willing to peek at the changes and provide thoughts or recommendations on organization? https://github.com/namesakefyi/namesake/pull/442/files
Most of the handiness of convex comes from thinking of mutation and actions as server actions, convex even pushes this with all their react-centric marketing.
They should allow running other functions from functions instead of you having to define same function name in 2 separate files.
@AlphaOmega This was my initial hurdle too. I think the intent of this change is that the model functions can be different from the API endpoints you expose. So it makes things more maintainable over time, because you don't have endpoints calling other endpoints.
I do think it would be helpful to make note of this in more places in the docs, especially for new users who are just getting familiar with Convex. The way that the tutorial is set up could make this clearer.
Yep, it's separation of concerns.
You'll see imports in the generated types but no routes will be generated from helper functions.
Then why not have a dependency injected or some other solution for the ctx param? Passing it as an argument doesn't seem like the best solution.
If you change functionality, you'll have to deprecate or rename convex functions, and that's why their great, because the client gets notified to through type gen.
Changing functionality but having the same name on client makes for bad maintainability
The convex team should focus on expanding on this feature and possibly, making it less tasking to run
For mutations specifically they should have errors bubble up to the top mutation so acid compliancy is maintained
It's explicit and portable, you can pass it around like a football. And it's fully typesafe. Lots of writing on what is and isn't best, but I've come to deeply appreciate things being very obvious in programming. The fact that ctx is just a value has made reasoning about it and scaling it's use across my backend a pleasant experience. Just my opinion as a user.
Depends on what you call typesafe, you still have to annotate it
ctx: ActionCtx for instance
That's actually a feature of the typesafety, I can say what kind of context my function expects, so I don't end up trying to run a mutation from a query context, or call an api without using an action context.
I'm curious on your viewpoint - is there something you've used in the past that you feel models the kind of ergonomics you expect?
with other backend oop focused frameworks ( not that im advocating for it, i find convex a joy because its not that ) Property Injection would achieve something similar, or a global importable ( much like the api )
But other than that ctx is fine, currently using it with a wrapper so my functions defined in model dont all have to expect a ctx
so something like
getCurrentUser = withQueryContext((ctx, ...args) => {});
where with query context is a function that returns another function
but i do feel that calling convex functions is more ergonomic
the import * is what hurts me the most tbh
just doesnt feel like ts to me
but its fine honestly, if yall know it to be better than im sure its better
Gotcha, that makes sense. A lot of folks have mentioned this, definitely not just you. I think I'm kind of in the middle at this point - I used to want to just call functions from other functions, but having a distinct api definition layer is starting to feel like a strength. But yeah, mileage varies.
It would be nice to have a Stack article! A primer on API/model organization like “here’s why to put your functions in a separate model layer, here are the benefits and drawbacks of this approach, here’s what Convex does to support this, here’s what we recommend”
I really appreciate the other Stack primer articles like this, they go a long way toward educating people about best practices
Another question I have is where to put my tests? For example, previously I had written unit tests for all my queries and mutations using convex-test. I can relocate the innards of the function into /model, and the tests still pass like before, but the actual logic being tested is elsewhere. Should I relocate the tests too, or is it still good to test the actual endpoint?
@erquhart what do you guys suggest about params, with having things in separate files, you do lose the type inference from the validator, should we create validators and export them from model/ files so we can Infer<typeof validator> and have total type equality?
I personally reuse validators from my schema, but otherwise I allow shared helper functions and Convex functions to be independently typed to reduce brittleness, eg., a Convex query may map directly to a single model function today, but if I change anything I have to update both. But yeah, you could definitely create and share validators if you prefer it.
In my experience the relationship between api endpoints and underlying functions can be pretty fluid over time.
yeah i also have a schemas folder that i use which also includes common validators so i don't have to explicitly type things out, but yeah its still a bit of a miss
and its equivalent in ts function form
which now that im thinking about it should be used here instead of duplicating ctx.db. but thats a small thing