No query type safety
Hello, I'm having trouble with why I'm not seeing proper type safety.
In my code:
objects
is type any
.
My schema:
schema/objects.ts
Convex dev is running and says everything is ready. What's going on here? It's all [x: string]: any
Imports
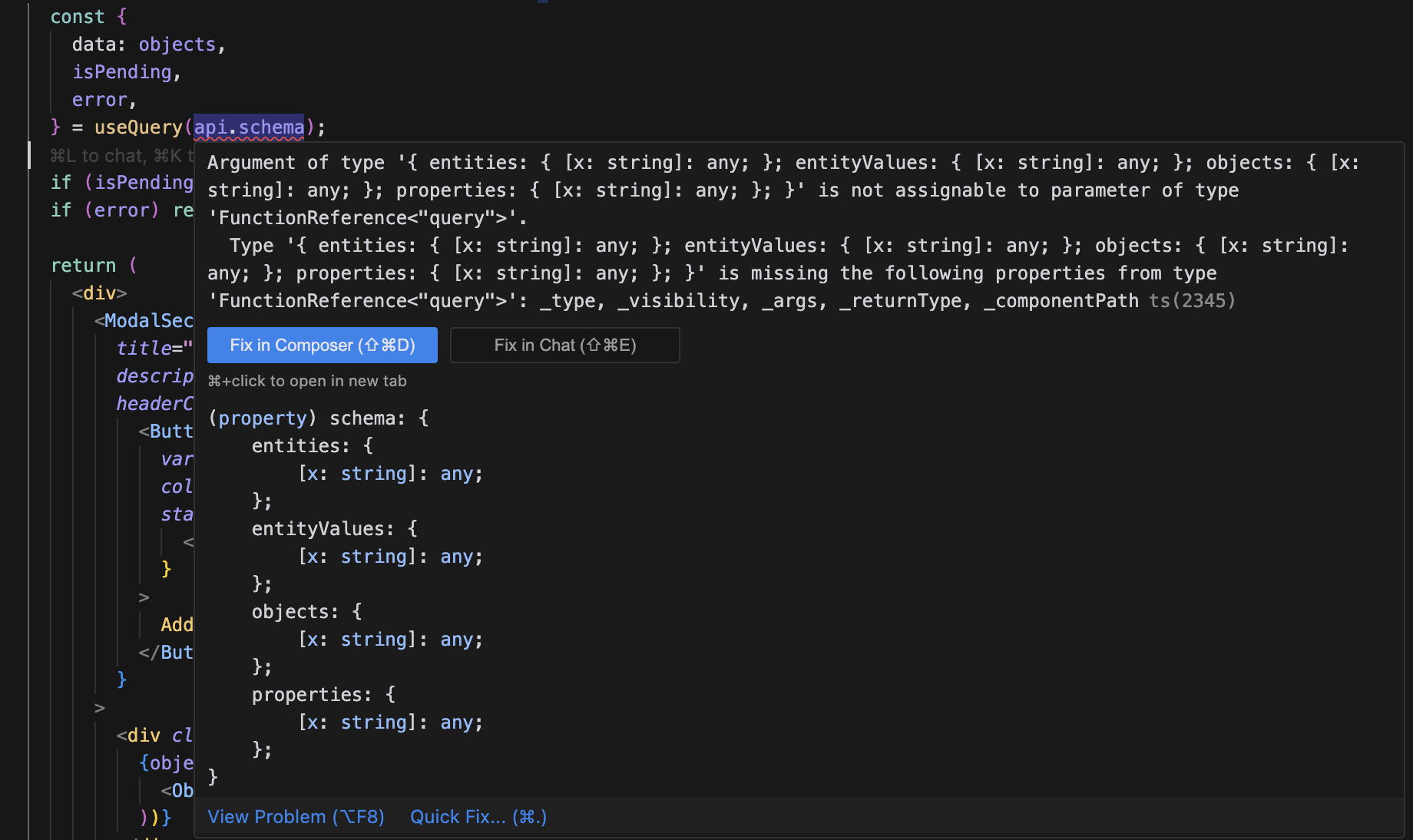
8 Replies
Sorry I suppose I shouldn't be destructure it, though I still don't see a type.
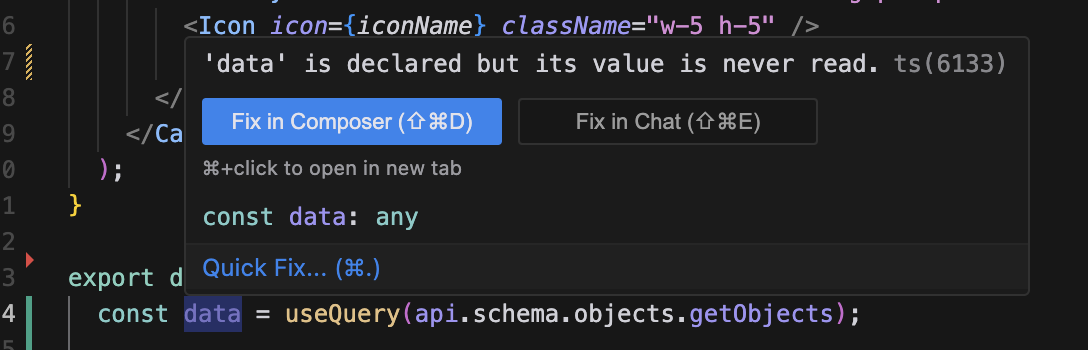
const data: Doc<"objects">[] = userQuery(...);
wouldn't this work?I'm surprised by the type
Promise<Doc<"objects">[]>
in the getObjects
query. My queries don't have any type here, it's all automatic.It would, though my understanding was that this should be automatic 🤔
Though this should actually be Taking a look…
I removed that bit; I'm not sure why I had that only for the object queries/mutation functions but not the others... my brain is fried. However, there are no improvements, and the other queries are still all
Doc… | undefined
right?
any.
schema/entities
Has type:
But in a component:
Has type any
for entity
.For clarity, this is another project I had in the past. Convex here had automatic typings 🤔.
Not sure what the difference is...
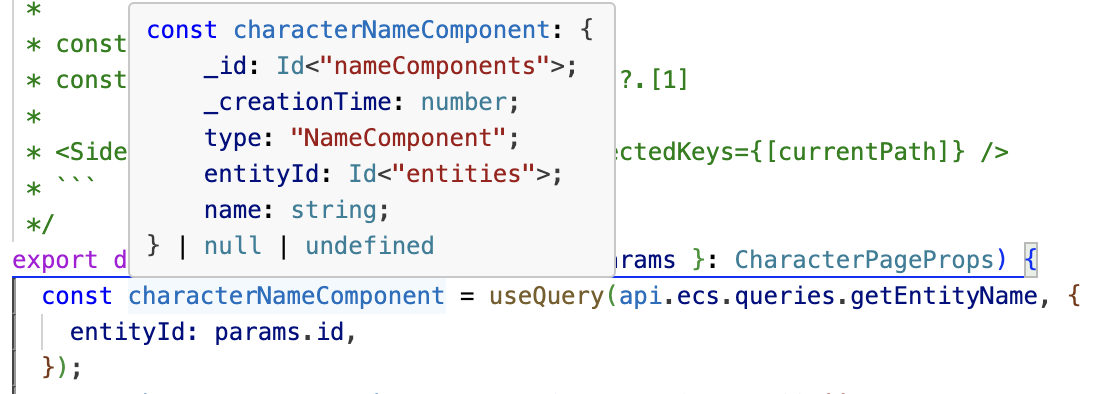
I'm noticing that in my previous project that I made a few months ago, there is no
tsconfig
file in the convex/
directory. The current one has one, though it's generated.
I deleted it, and the type safety is working now. This doesn't seem right: "You can modify it, but some settings required to use Convex."do you know where the
part of that tsconfig came from? I don't remember seeing it in any convex tutorials
I added it for easier imports. I put the config file back (including the I do wish it were possible to use paths though 🤔
Nevermind, got it to work. Just have to configure the same path key to the relative values to each
paths
I added), and the typings are working now. I'm quite confused. This isn't the first time I've restarted my IDE, either.
Well, I'm not sure what I did to be honest besides deleting that file and adding it back in...
Thanks for everyone's help; hopefully, it doesn't break again.
Oh, actually, the paths thing was breaking it. Huh, it seems like the tsconfig
from the overall project cannot reconcile with the paths configured in the convex tsconfig
. I switched the imports in the convex schema back from relative to @
, and it broke 🥲. Thanks Lee!
tsconfig
, in both files.