Dynamic query builder in convex
I'm trying to do a dynamic query based on conditions
schema.ts
I get a huge error, sorry for the spam
and all the args need to be marked with
!
8 Replies
Thanks for posting in <#1088161997662724167>.
Reminder: If you have a Convex Pro account, use the Convex Dashboard to file support tickets.
- Provide context: What are you trying to achieve, what is the end-user interaction, what are you seeing? (full error message, command output, etc.)
- Use search.convex.dev to search Docs, Stack, and Discord all at once.
- Additionally, you can post your questions in the Convex Community's <#1228095053885476985> channel to receive a response from AI.
- Avoid tagging staff unless specifically instructed.
Thank you!
What happens if you ask chatgpt to rewrite your code with the pattern i posted in the other thread? It gave me a good result when i tried it
Can't paste because it's too long
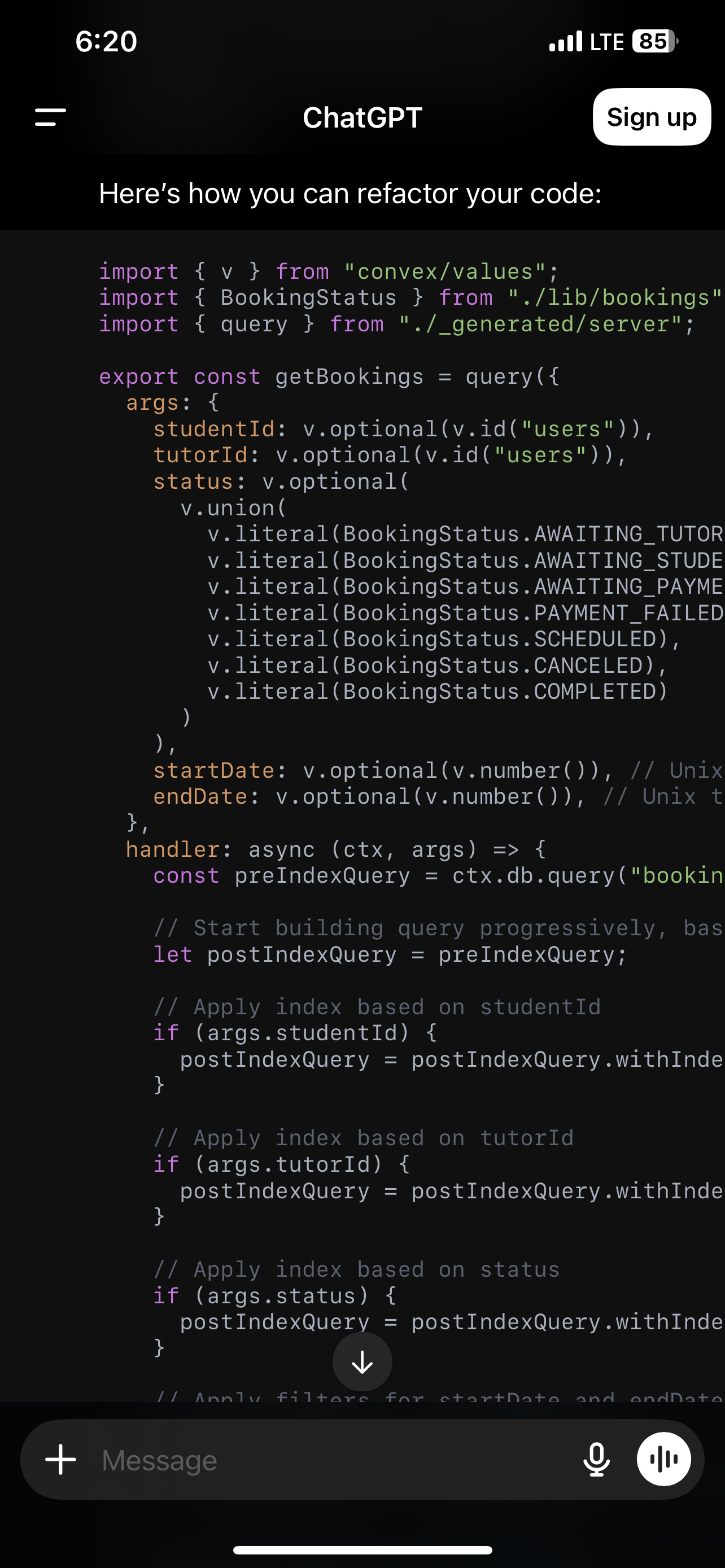
The important part is you're splitting up the query construction into stages, so that you can prove to the type system that you're only using a single index.
Okay actually chatgpt can't figure it out
Maybe claude can do it? Chatgpt is refusing to follow the pattern
I also see your thread in #ask-ai . If the pattern is so hard to figure out, we should make it easier with helper functions
Thanks @lee, I tried gpt too and the pattern was refused. Do you have some posts to advice me this? It's a common thing in Read.
I would try to do it by hand. The pattern should work
I don't think we have posts that discuss the solution, although we could write one
You could @lee, it would be appreciate it.
I wrote this up. It's not polished or reviewed, but might help https://github.com/ldanilek/query-cookbook/blob/main/DYNAMIC_QUERY.md
GitHub
query-cookbook/DYNAMIC_QUERY.md at main · ldanilek/query-cookbook
Contribute to ldanilek/query-cookbook development by creating an account on GitHub.