Supposed to return ids in random order but mostly does one image
I'm trying to pull down 5 document entries in my "levels" table and it seems to only ever return the ids in the same order most of the time. Every once and a while and sometimes when I have to refresh the npx convex dev server, will it then pull a different image. Is my code wrong?
6 Replies
Thanks for posting in <#1088161997662724167>.
Reminder: If you have a Convex Pro account, use the Convex Dashboard to file support tickets.
- Provide context: What are you trying to achieve, what is the end-user interaction, what are you seeing? (full error message, command output, etc.)
- Use search.convex.dev to search Docs, Stack, and Discord all at once.
- Additionally, you can post your questions in the Convex Community's <#1228095053885476985> channel to receive a response from AI.
- Avoid tagging staff unless specifically instructed.
Thank you!
This is where i call the functions
Okay so an image finally changed. Then when I refreshed again the next one was cached
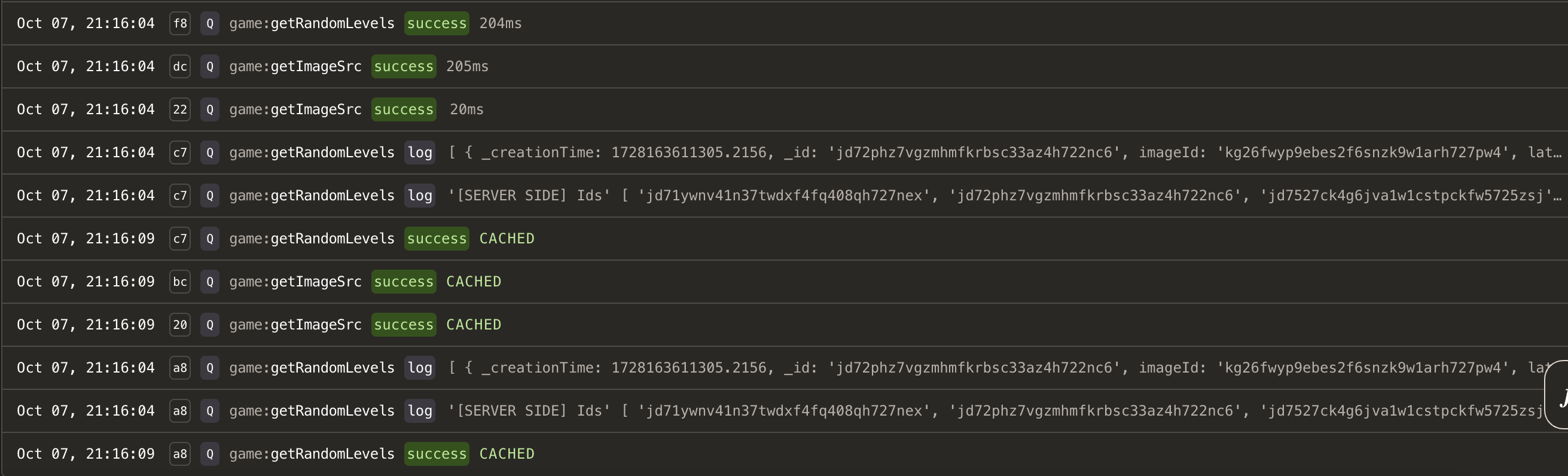
how do i not cache them?
queries are cached when their arguments and the documents they read are the same. You can "bust" the cache by passing an argument which you can change.
this is a pretty good description https://www.keycdn.com/support/what-is-cache-busting
KeyCDN
What is Cache Busting? - KeyCDN Support
Cache busting solves browser caching issues by using a unique file version identifier to tell the browser that a new version of the file is available.