Auth Callbacks cannot use indexes when making a db.query()
I am attempting to use an auth callback to populate a table after user creation. This works, however, I need to query this table first to avoid creating duplicate records. Typescript does not accept any queries that include a withIndex(). Unclear whether I am doing something wrong or not?
Example:
Output:
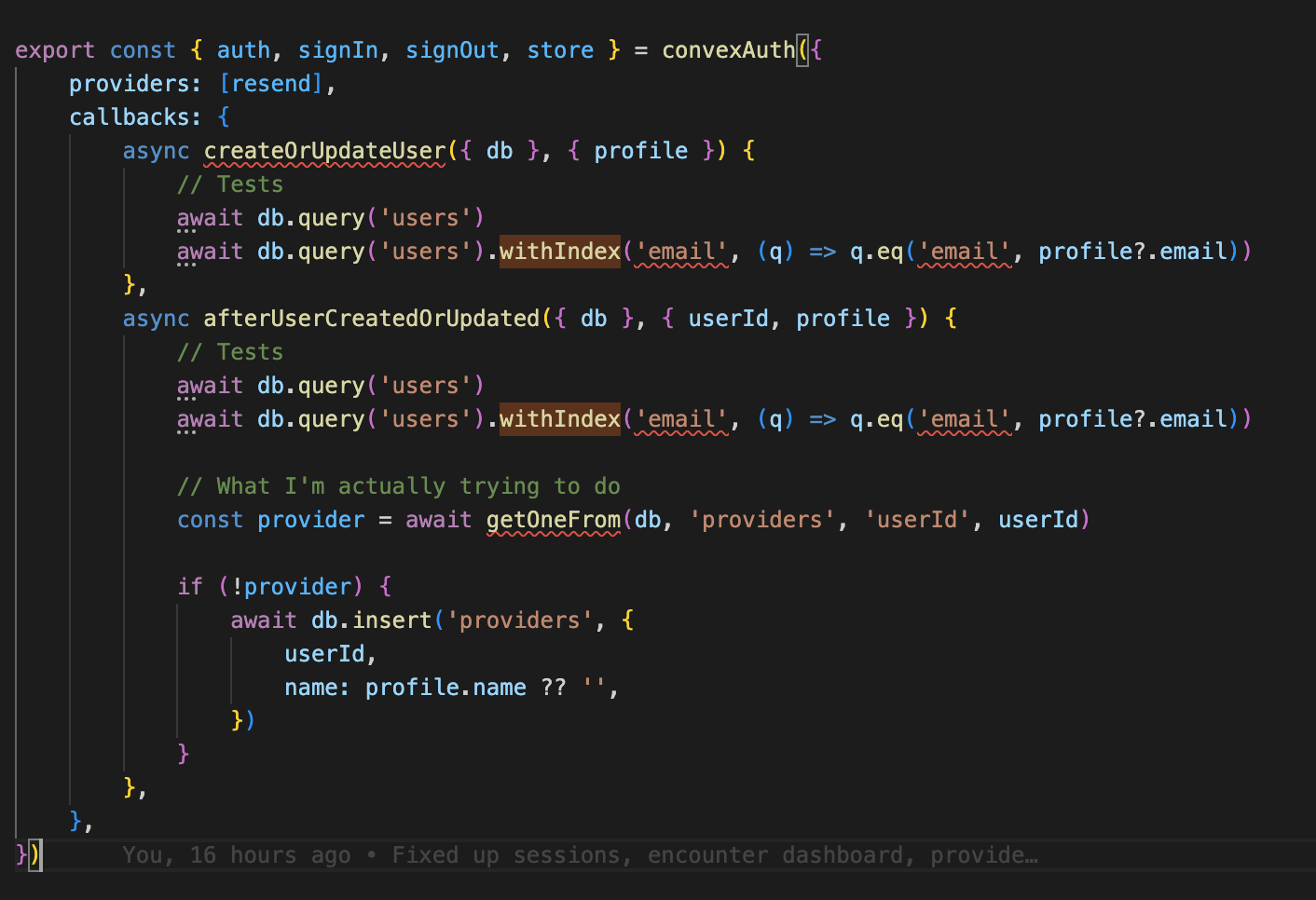
2 Replies
Agree this looks like a bug with the types.
We've currently typed it so the
ctx
/ db
in those callbacks doesn't know about your schema. To work around this, I'd add a type annotation ctx: MutationCtx
(where MutationCtx
comes from your _generated/server
file) to the first argument of those callbacks@sshader thank you! that helped