Help running an internal query
I'm trying to run a query from my Node backend, but Convex doesn't seem to be including my function in its generated output. I've verified
npx convex dev
is running.
Here's what I'm doing:
In packages/convex-server/convex/server/internal.ts
, I define the internalQuery
:
In packages/convex-server/convex/server/actions/index.ts
, I define the internalAction
:
In my nodejs server, I try to call it like this:
I'm getting 2 errors - the 1st is a Typescript error when I try to return data in the internalAction, the 2nd is that getLastReadMessageByUserIdAndSectionId
is not available in api.server.actions.index
. How can I resolve this?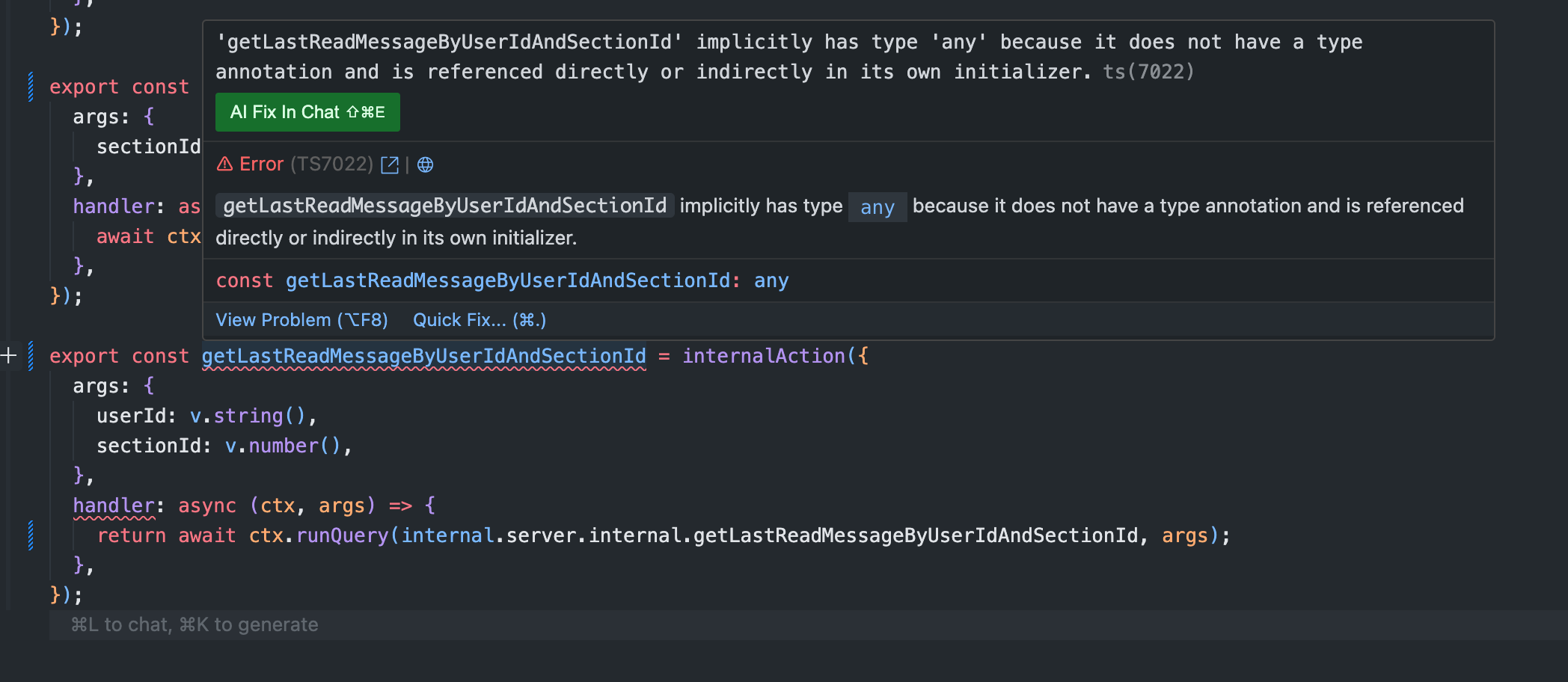
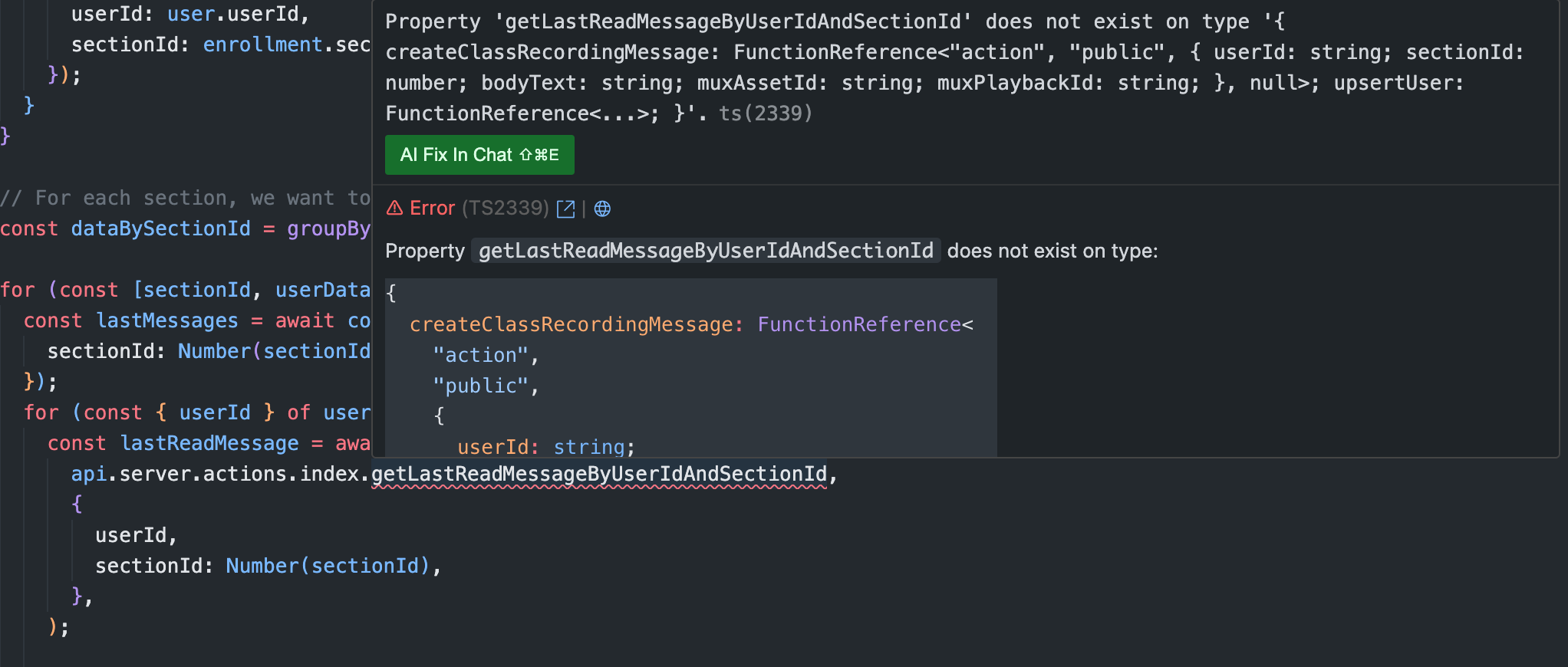
10 Replies
1. Circular reference errors are a known issue. There's a workaround described in https://discord.com/channels/1019350475847499849/1093388424582529104/1093516566127190118
2. internal queries/mutations/actions can only be called by other convex functions (or the dashboard or CLI). If you want to call it from a nodejs server, you can make it a non-internal action and authorize it with a shared secret
So instead of using
internalQuery
, i should use query
, and instead of internalAction
, I should use action
?right. And you can ensure that only the node server can call your action by having an argument which is a shared secret
Hey there, I'm not seeing a circular reference error, but for some reason I'm still running into the same issue when I try to use a query.
the functions w/ the green arrows are working correctly when I try to access them from the convex client, but the red arrows (the queries) are not.
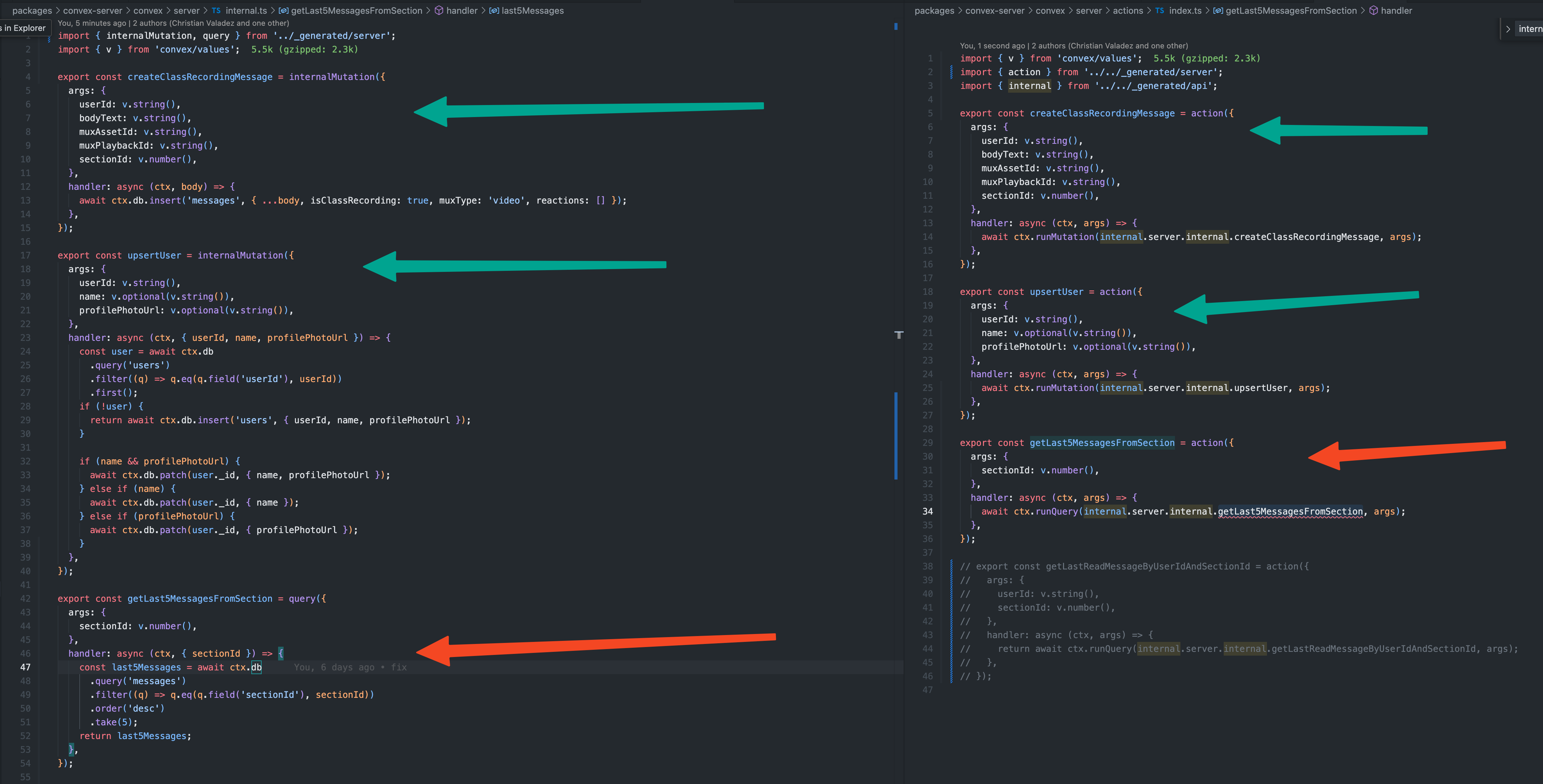
do those functions show up in your dashboard?
that's a great way to make sure they're actually registered in your deployment
the green arrow ones do, but the red arrow ones don't.
npx convex dev
should push them, right?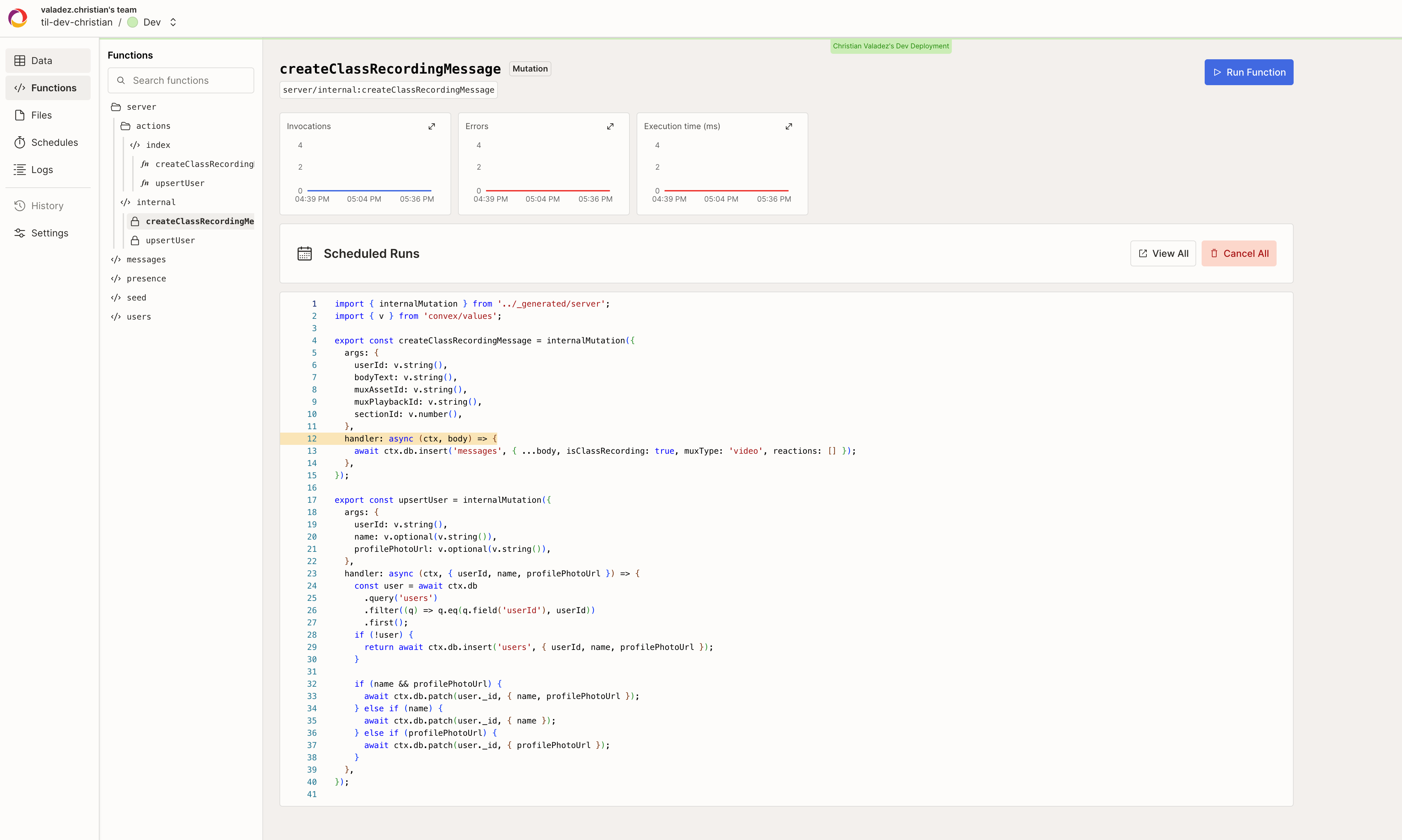
yeah -- do you have any output from
npx convex dev
running in the background?
seems those functions aren't syncingI just re-ran
npx convex dev
, and it looks like they're there now. But, the TS still isn't working - do you know if there's a way to fix this? It's saying that getLast5MessagesFromSection
does not exist on internal.server.internal
.
Alternatively, is there another way to run that query from the convex client in node, without wrapping it in an action
call?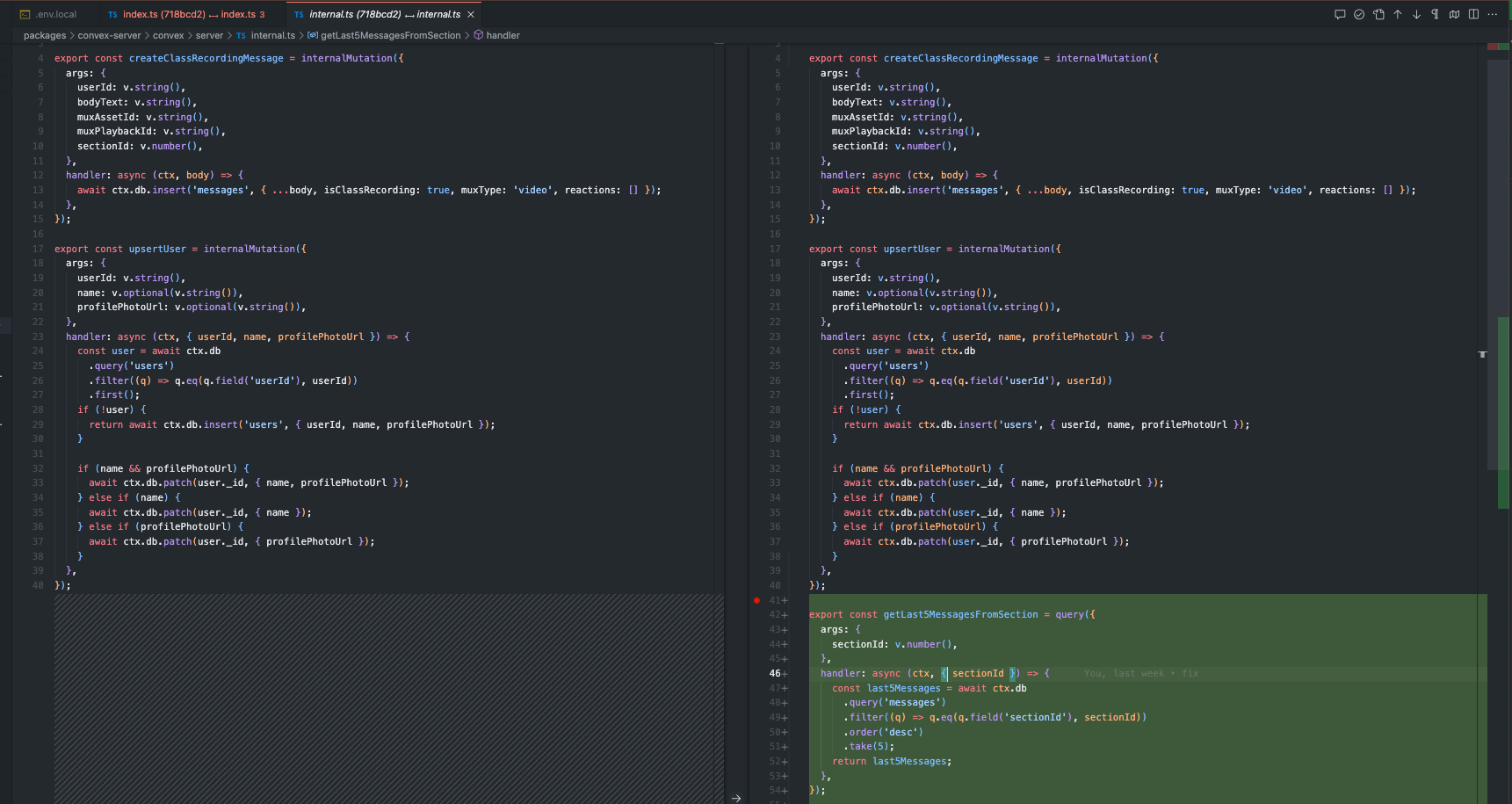
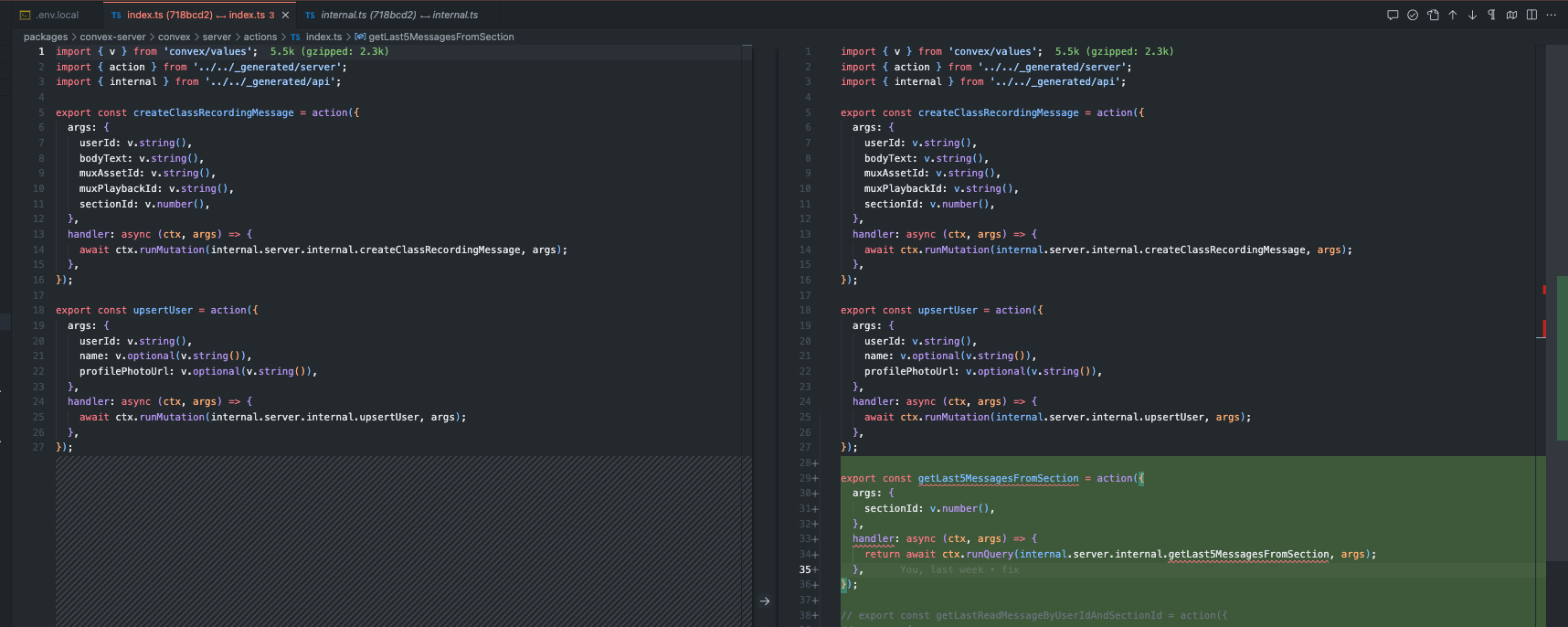
Curiously, the mutations work just fine...
Hey there, was just wondering if you had any insight into why TS may not be generating correctly?
getLast5MessagesFromSection
is not an internal query or mutation, so it exists on api.
, not internal.