Get Price highest to lowest with pagination query
I am currently designing a filter which can take in " sorted by " and apply it to a convex query, there are 4 options,
Sorted by Newest (newest to oldest)
Oldest (oldest to newest)
Price (highest to lowest)
Price (lowest to highest)
There are also additional filters such as categoryId of the products and min / max prices
The paginated query looks like this:
"desc" meaning from Price highest to lowest
"asc" meaning from Price lowest to highest
However this does not provide me with the intended result as it only sorts after the pagination. I have tried using withIndex("by_pricing") but it gives an error saying .withIndex is not a function. Please help! Thanks
14 Replies
withIndex
needs to go before the filterswith this updated code, i no longer get it sorted by price, instead it sorts by creationTime
change
res.withIndex("by_price");
into res = res.withIndex("by_price");
It gives an error under res saying
Type 'Query<{ document: { _id: Id<"post">; _creationTime: number; title: string; src: string; description: string; location: string; pricing: number; categoryId: Id<"categories">; userId: Id<"users">; likes: number; interactions: number; }; fieldPaths: ExtractFieldPaths<...> | "_id"; indexes: { ...; }; searchIndexes: {}; ...' is missing the following properties from type 'QueryInitializer<{ document: { _id: Id<"post">; _creationTime: number; title: string; src: string; description: string; location: string; pricing: number; categoryId: Id<"categories">; userId: Id<"users">; likes: number; interactions: number; }; fieldPaths: ExtractFieldPaths<...> | "_id"; indexes: { ...; }; searchIn...': fullTableScan, withIndex, withSearchIndexts(2739)
ok yeah the types aren't right. i would do
got it, thank you so much ❤️
sweet! btw if you're interested in writing very complex filters, you can check out https://stack.convex.dev/complex-filters-in-convex
Using TypeScript to Write Complex Query Filters
There’s a new Convex helper to perform generic TypeScript filters, with the same performance as built-in Convex filters, and unlimited potential.
I also require title to be part of the filter, problem is i am unable to use withSearchindex to do the fuzzy search on all documents with similar titles, currently i am using
However, this only works if the title is EXACTLY the name parameter i search for, how do i work around this?
why do you can't use withSearchIndex?
e.g. searching all posts by title, then manually sorting those by price? presumably the title results wouldn't be so many that they'd need to paginate on price?
im trying to combine all the filters into one function
i cant use searchIndex together with withIndex
for example if the user types Property, i want to find every post with property inside the title along in the paginated filter query above
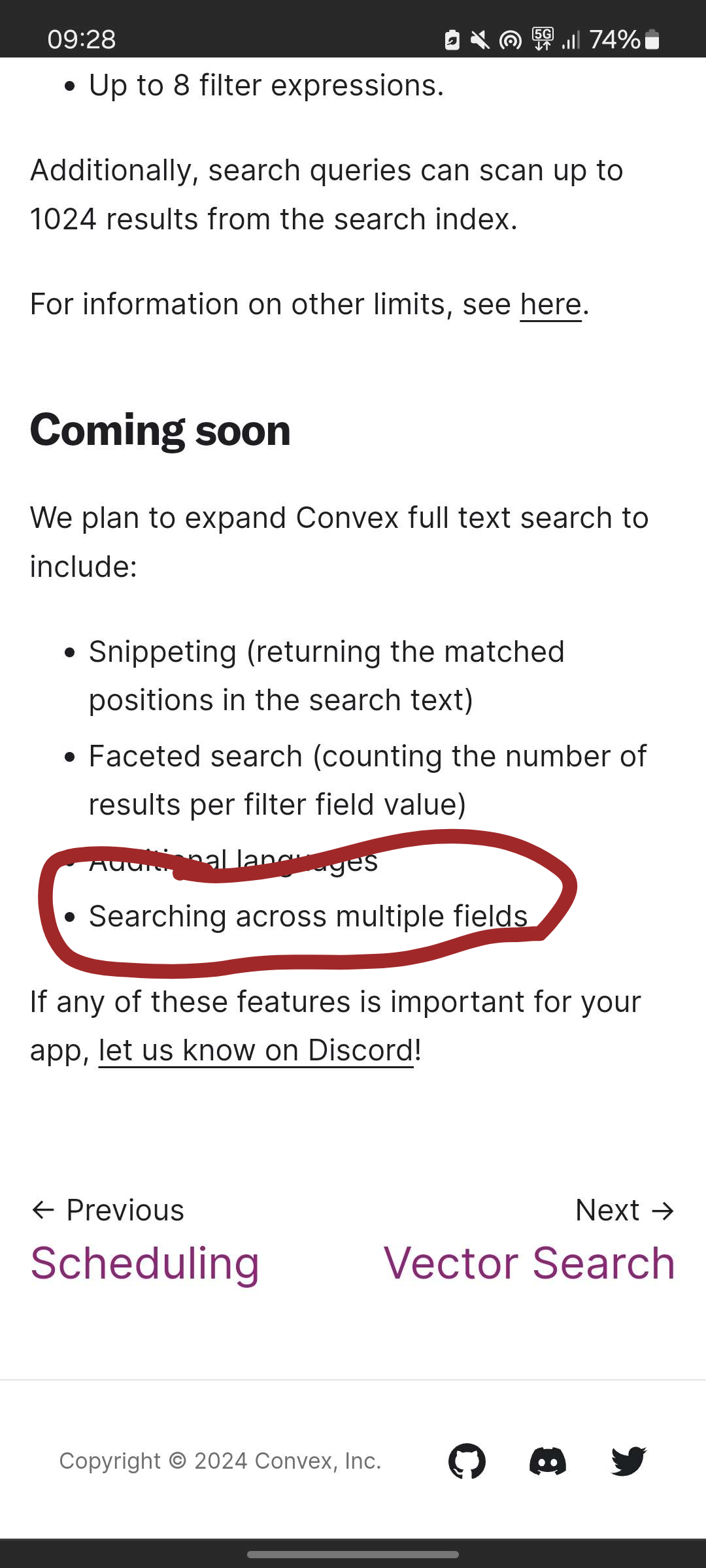
Seems like it's coming soon
searchIndex can only return items sorted by relevance, so it doesn't sound like what you want. I would use the withIndex and implement fuzzy search in a custom filter (https://stack.convex.dev/complex-filters-in-convex)
Using TypeScript to Write Complex Query Filters
There’s a new Convex helper to perform generic TypeScript filters, with the same performance as built-in Convex filters, and unlimited potential.