Custom Auth integration with Privy
Hey everyone! I'm trying to get a custom auth integration working, but can't quite crack it.
I use Privy.io web3 auth and this is what I came up with so far:
Here,
authenticated
always returns false
even though console.log('Privy authenticated?', authenticated);
returns true
after I verify the user token. The isLoading: !ready
works as well. Any suggestions?6 Replies
To add more context, I'm following the debugging steps and I get the
Authenticated
status in my browser with the correct token.
The JWT decoder looks correct as well, it all matches with the Settings > Authentication
menu in Convex.
My authConfig:
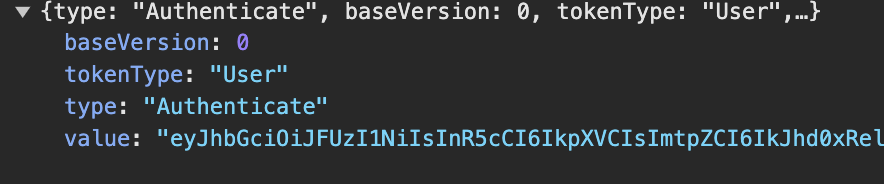
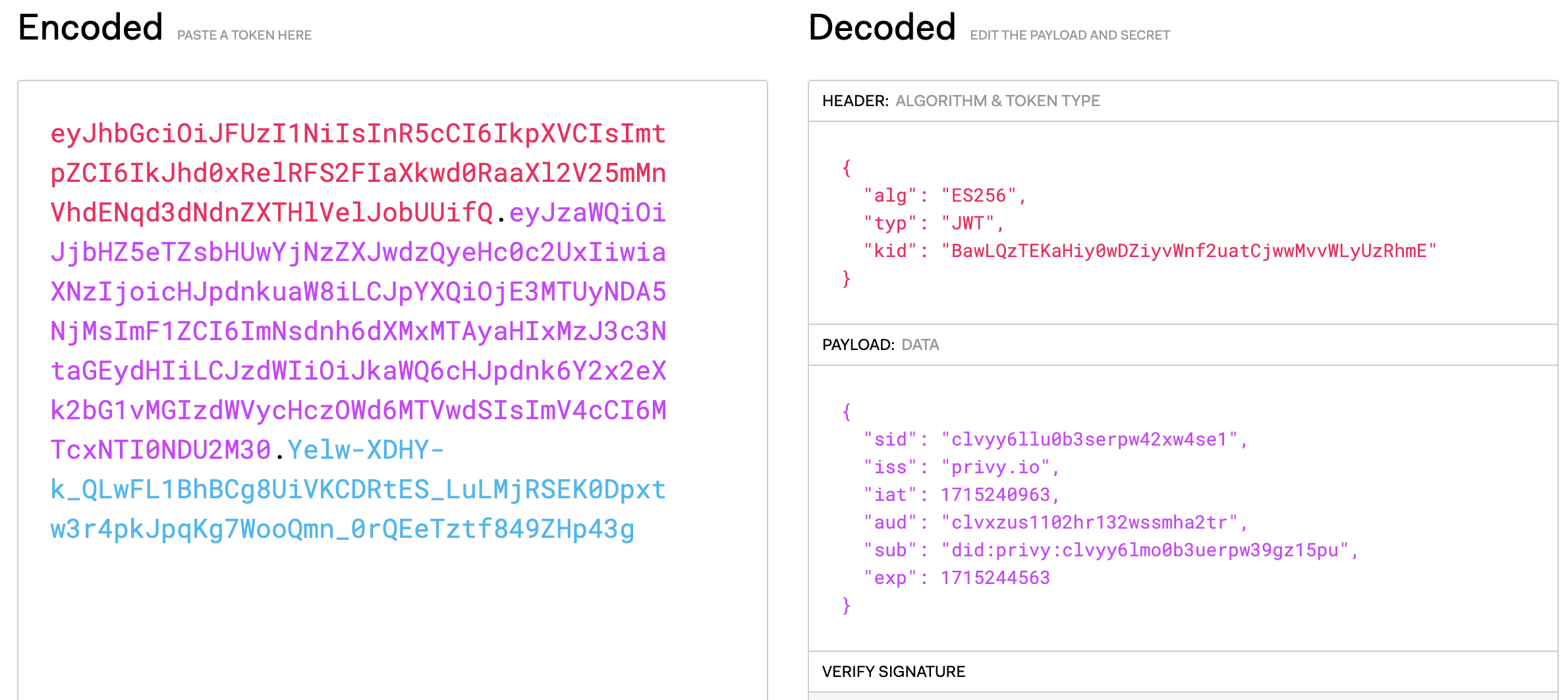
What error are you getting? (you should be getting AuthError message in the websocket messages).
I'm suspicious of the
iss
not being a full URL.In my ws I get "Could not parse as id token".
In my Privy dashboard I have an endpoint like https://auth.privy.io/api/v1/apps/clvxzus1102hr132wssmha2tr/jwks.json but it doesn't work either, exact same behaviour and errors.
The Convex backend is following OpenID strictly. Usually the endpoints for JWKS are under .well-known/.
I would guess this JWT setup doesn't follow the OpenID spec.
Understood, thank you for your help, Michael!
Reaching out to their team.