Unsure about right pattern when following zen of convex for actions
I'm trying to follow the concepts from the zen of convex. In this case i wanna create a new user from the client.
I go ahead and make a mutation, that i can call from the client, like this example below:
Am i on the wrong path here, i'm assuming it's best to create my hashed password using a action running on the server, but finding it a bit hard to follow zen of convex here.
14 Replies
I think the zen-of-convex way to do this would be to do it all in the mutation; hashing a password is just compute, not interacting with an external system. So you shouldn't have to break out into a scheduled action. Is this because the Convex runtime is missing some hashing functionality you need?
Deleted previous reply, as i got confused. So i basically cannot do this in a normal mutation, which is why i'd wanna use an action:
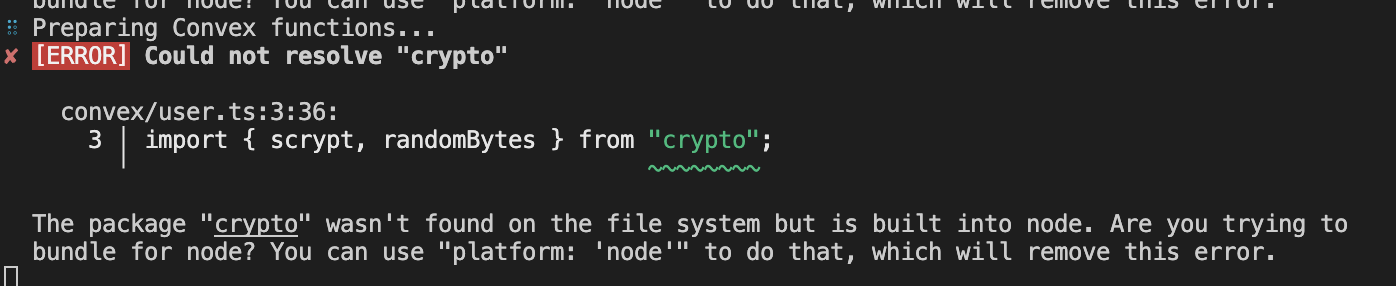
Yeah looks like
scrypt
is not provided by WebCrypto, the crypto that is build into the Convex runtime https://developer.mozilla.org/en-US/docs/Web/API/Web_Crypto_API
You could use https://www.npmjs.com/package/scrypt-js
I found this helpful reading https://gist.github.com/chrisveness/770ee96945ec12ac84f134bf538d89fbYeah. So basically to give a little context here, i'm just trying to learn about authentication - trying to build a small authentication system with pure convex.
I know lucia auth provides some packages, and helpers for all of this. But i'd like to see how far i can get by just using convex and whats available to me.
as in using no npm libraries? cool!
Awesome, thanks for linking. I'll take a look at that!
IF the goal is just to hash a password https://developer.mozilla.org/en-US/docs/Web/API/SubtleCrypto/digest looks good
Yeah exactly. I mean my whole point of wanting to learn about it, is that i eventually wanna try and cut clerk (although i love what they provide)
but i mean i'm very curious about auth
so i wanna see how far i can get on my own, and i feel like that's also where im gonna learn most, since it 4 sure aint easy stuff
atleast very different from my day to day
seems to work
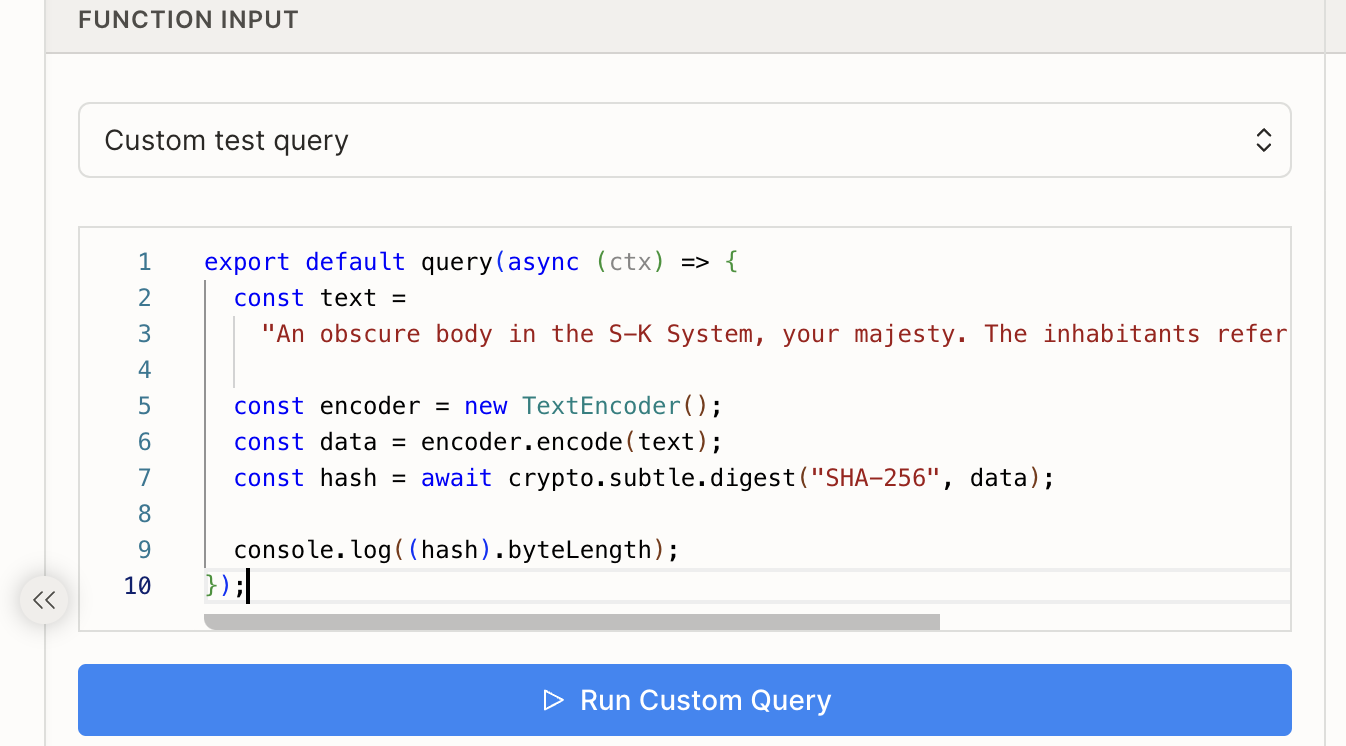
Yeah, then my next step is authenticating the user, and this is what i've gotten so far:
Still very early on with this project, but i'm keeping it 100% open source here, hopefully at some point reaching something that can be used. Then comes all the security improvements haha, but one step at a time
GitHub
GitHub - oscdot/convex-auth
Contribute to oscdot/convex-auth development by creating an account on GitHub.
awesome!
I'm glad you say that. It's fun so far, learning a lot of stuff
Thats awesome! looking thorugh the links u sent