How can I use timeout in mutation?
I have set up the following configuration using Clerk webhooks:
1. When an organization is created, a Document of type "workspace" is also created with the same data.
2. When an organization membership is created, a Document of type "workspace membership" is generated.
The issue arises when creating a workspace, as both steps 1 and 2 occur simultaneously. During the process of creating a workspace through step 1, if step 2 is triggered before the Doc<"workspace"> is created, it results in an error because the Doc<"workspace_membership"> cannot be created without the workspace existing.
To address this, I implemented code that uses a timeout to wait until the workspace is created before proceeding with creating the workspace membership.
However, using a timeout within the mutation has led to errors. Is there a better way to handle this situation?
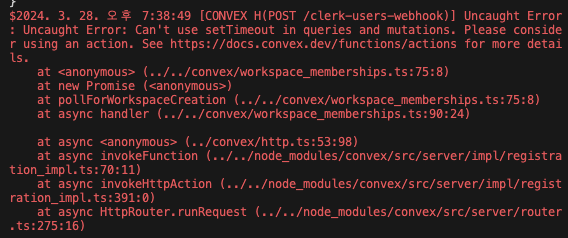
6 Replies
This is the code.
I would avoid the timeout approach as it has no guarantees. There are definitely options, though, but I need to understand your context a little more. I'm guessing you're using Clerk's org components and getting a webhook when an org is created. When is the function above called?
If a user creates a new organization through your Clerk component, and the user is made a member of the Clerk org as a part of that process, I would have the webhook get the users in the new org from Clerk and create the records necessary in your Convex tables. Then you just need to have your UI show a loading state until the records are created via webhook.
Again, that's just a stab without knowing the details of your implementation.
(another option close to the timeout approach is to have the mutation schedule itself with a little delay)
Just realized
setTimeout
not being available in mutations is your core issue, this is what I get for trying to help on mobile lol. Lee's answer is likely what you're looking for.btw even if setTimeout did work in mutations, you probably wouldn't get what you expect because mutations are transactions. So if you read a document twice, you'll always get the same result no matter how long the mutation takes.
I agree with @erquhart that this should not require waiting. Your issue is you're getting a request A and request B, in arbitrary order. But if B comes before A, it cannot proceed. So if B came first, I would store all the required information from B into the database, and when A comes in I would check whether the info from B was stored, and if so perform A and then whatever B would have done.