Adding tags
Hey there, I'm trying to make tags for my project, basically every post has tags and then you can filter through those tags which will show all posts with that tag. Though every time I try and filter through it I get nothing back, It's probably a Syntax issue but I was wondering if I could check if an array includes something ? Here's my code:
I'm using Next 14 btw and this is the code for it:
So I'm wondering if there are any solutions to this. Thanks in advance and I apologize if this isn't the right channel for this.
15 Replies
Result from console
https://discord.com/channels/1019350475847499849/1138800227416014951 has some good info
and if you are willing to have your tags be a space-separated string, https://discord.com/channels/1019350475847499849/1207072218744492072 would be a good option too
Alright , so something like this ?
@ian Or would this still be incorrect if you know
First time doing something like this so, sorry if it's obvious to make
Take out the tags array and add another table called “tags” that matches your ID in the postTags table. Create a tag document for every name you want, then for every post, add a document to postTags with the post’s ID and tag’s ID
Something like this?
And what would I need to change here? I'm assuming a forloop and I've tried but no success so far:
My post action as well
I don't see you using the
args.tags
yet, and I think tagId: v.string()
isn't needed in the tags table
if you can pass up tags by their ID:
otherwise if you pass up the names, something like:
...
Just wrote that in Discord, so apologies for any syntax snafoosHey there, I tried this out:
But tags aren't being saved in the tags table nor the postTags table despite me passing in them in my form. They do however get saved in the posts table which is the same as before.

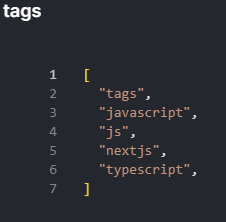
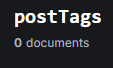
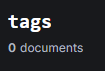
And this is my createPostAction
@ian If you could look into this it'd be super helpful as I cannot figure out what's wrong
I think your code hasn’t been updated if it’s still saving the tags in the table. You probably need to clear that table since it has the tags values that don’t exist anymore
And it should be
tag?._id ||
I don't know what I changed but I can confirm that it works, I think it was this that caused the difference and it works! Just wanted to ask how I would filter now through these as the filter I've written doesn't work 😅 and was wondering if it's possible or just a syntax error:
using .take(5) at the moment until the filter works.
I think what you want is to get all of the tags by the ids you now have
There are good blog posts on stack about relationship structures with code examples. If you could drop a link here to the one, that is the most useful, I would appreciate it.
Yes, thanks. In particular, what you've made with the postTags table is a "many to many" relationship with a "join" table.
This is what I was able to make , and it is functional from what I've noticed
So now, if I wanted to get all posts by x tag name e.g. /tag/typescript , then I'd need a one to many , correct ?
Nice! 👏