Possibility of making a object validator all optional for use when patching fields
Say i have this:
In this case, i cant just pass what i want but need to pass all values that aren't already optional
I might have missed some smart way here. But this is a solid wall i hit very often.
8 Replies
How are you typing
updateSubmission()
, that's a server function yeah?If i understand your question correctly. updateSubmission() is in this case:
You can write a function that returns v.optional wrapping each validator in an object. Getting the types right is a bit tricky but doable. something like
type Opt<V> = V extends Validator<infer T, any, infer FP> ? Validator<T | undefined, true, FP> : never
then the overall object is something like
just throwing some things out there. If you get something working please share. I've been meaning to write a partial(fields)
utility function that returns such a thing with the right types
I have a Table
helper in convex-helpers
that gives you fields with and without system fields and a doc wrapped in v.object
but it could compose well with a partial utility too
one caveat is you might not want to wrap optionals: v.optional(v.optional(v.number()))
but I'm not sure if that would be a problemCool thanks Ian. I'll try and fiddle around with it and report back!
So i might be on the wrong chat completely, but something in the area of this is what im trying to. The types gets lost in the args though, and havent figured out yet why.
The bookmark fields comes through as unknown
I'm still learning advanced typescript. Not really there yet. But this is what i've gotten so far
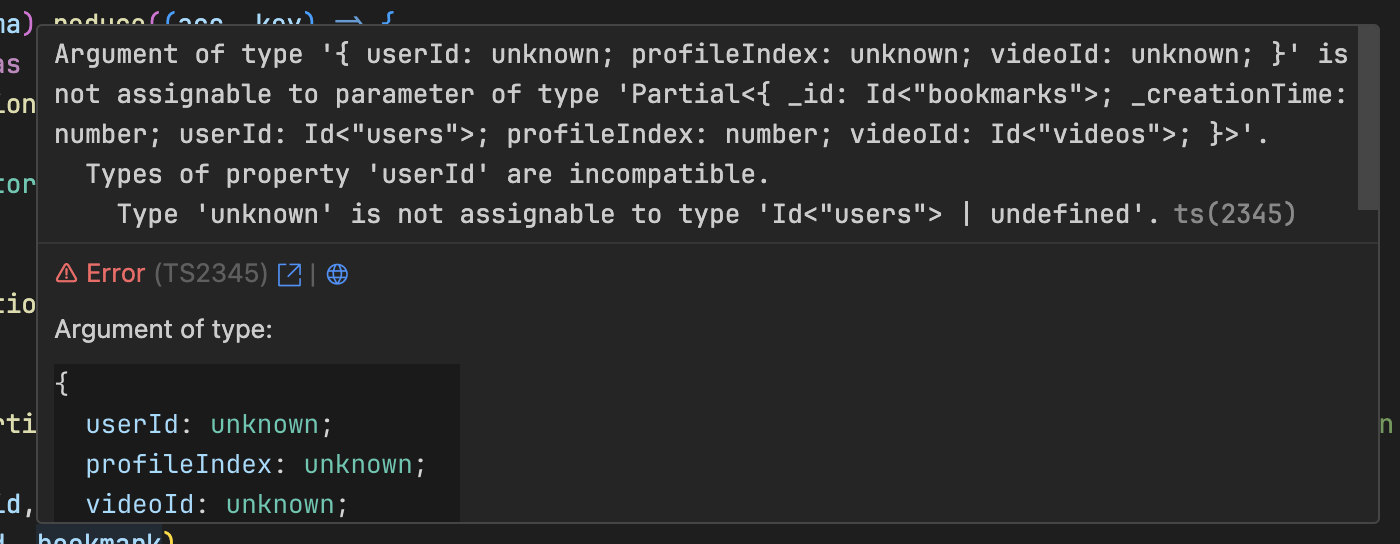
^ what the bookmark from args comes through as
Seems like also doing reduce, isnt the way about it here since its having a tough time inferring the keys . Dunno
I know that this is not the answer you're looking for, but I'd suggest to explicitly define the function argument validator - otherwise as your schema evolves, you might make a field editable from any client without realizing it...
That said, you'd want this for
OptionalValidator
:
Thanks a lot Michael. I can see how what you mean and how that could be an issue. Thanks for the help with the OptionalValidator I’ll try that out when I have the opportunity