Confused with passing additional arguments to paginated query
Hello,
So i'm trying to play around with passing paginated messages, and when i pass additional arguments to the query, it breaks something.
I'm only getting this error, and loosing my types - but other than that i get the messages with the authors and can display them as expected.
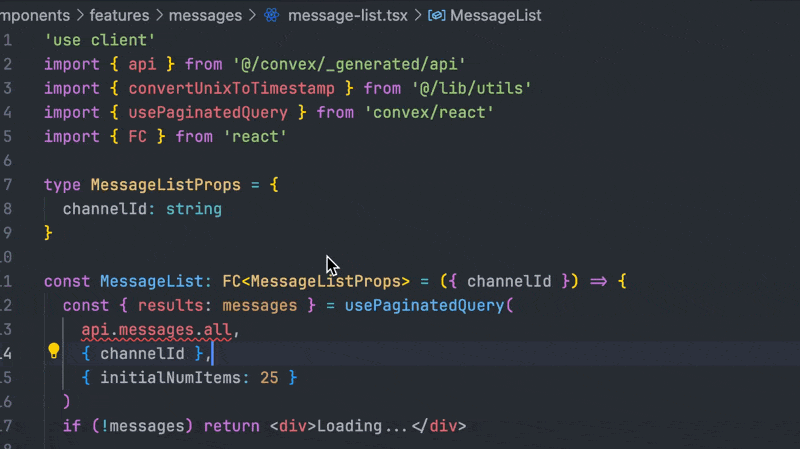
7 Replies
I just tried backtracking a bit, and seems like the issues lies in the withIndex, because i only want the messages that has the correct channelId
If i remove withIndex, everything flows through without issues. I cant seem to put my finger on why its breaking the query reference, atleast i think thats whats up
This is because of the
if (!channelId) return;
line: this query can return undefined (translated to null on the client), which doesn't fit the paginated query signatureOhh darn it, is that it haha :noice:
I'd use "skip" on the client to avoid this... oh right, we haven't published skip for usePaginatedQuery yet! It's coming!
I think you you can use a nonexistent channel id by just removing that line
should return zero results
Yeah was about to say that, it's prob not end of the world removing that check.
Thanks for clearing it up, i def ended up overthinking what was happening here
You could also move this usePaginatedQuery hook into a component that is only rendered if you have a channelId, we've talked about this workaround before so you may be tired of hearing about it when "skip" would be more convenient. Don't worry, it's coming!
Thanks for posting your code, made this much easier to figure out!
Your most welcome. I think the reason i put the check there in the first place, was that
.withIndex('by_channel', (q) => q.eq('channelId', channelId))
will complain that channelId cant be null
And its required to be a Id<"channels">
If i just do
That works, but results in the usePaginatedQuery isLoading just ends up returning true forever. But i'm asuming there is no way around this for now?