how to populate form with default values?
hi team, when i have the following code, it doesnt populate my form with current values in the db because it's undefined for 3 seconds before the values show up.
by then, the defaultValues in the useForm have already been populated with empty values. does anyone know how can i fix it?
in other words, how can i call the useForm hook after it finishes loading? thanks!
8 Replies
Guessing you're using react-hook-form, they give this approach for async default values in the docs:
In your case you could make a promise that resolves when your query comes back
Hmm trying to think of how to do that idiomatically in this case
I think I'd wrap your form along with
useForm
into a separate component, and skip rendering until myShop !== undefined
.
Convex team may have a better idea 🙂if you do
const convex = useConvex();
then you can call convex.query(api.myShopFuncs.getMyShopProfile)
which returns a promise. Then you can use @erquhart 's async defaultValues
idea to await the promises and get the valueswow thanks! looks like it's my lack of familiarity with the react use-form hook after all. anyway, i tried doing it but still running into
undefined
when it first renders. i should probably move the useQuery
one level up the component. do y'all have better suggestion?
That's pretty weird,
should never return undefined. It could possibly return
null
if the query function returns undefined.
Could you try changing the console.log() to something like
just to confirm?ahh you're right. my bad, it's because the
anyArgs
passed into the function is undefined too... 😂
looks like the only way i can get around this is to wait for the values to show up before im able to pass them
thanks all!! 🙂
on a side note, is it possible do sth like Awaited<ReturnType<api.anyApiFunction>>
?Totally, this almost works as you've written it
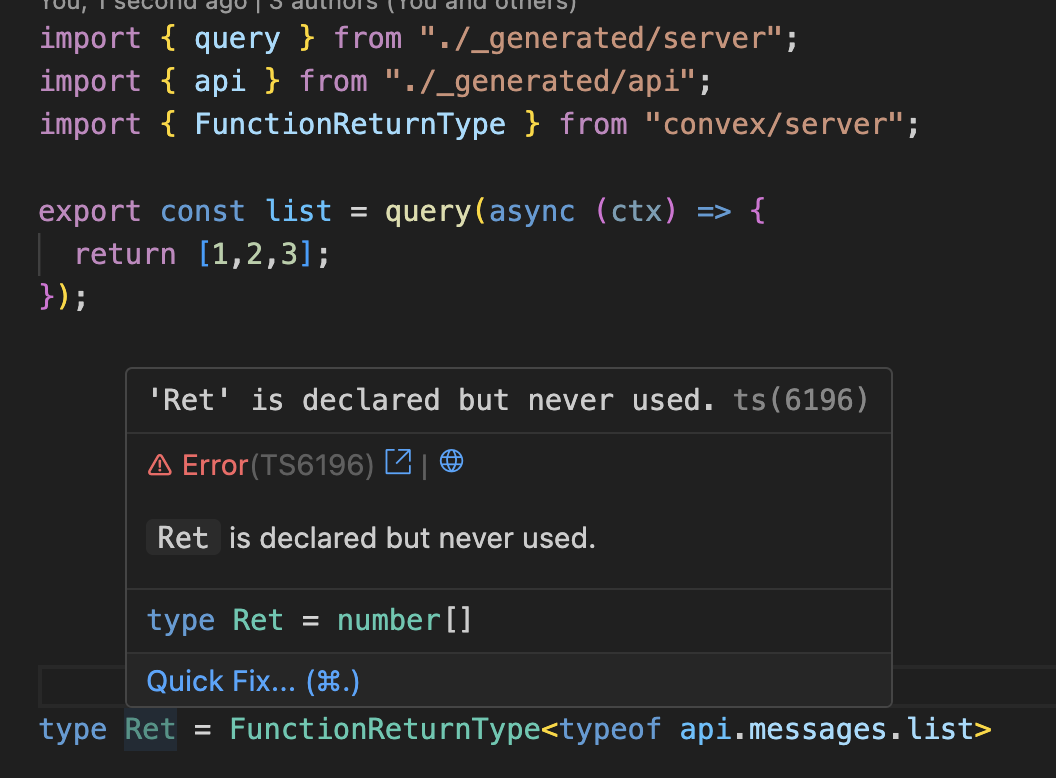
You don't need the
Awaited
if you get it off of the api
object, return types have already been transformed there.