13 Replies
undefined || [] || null
is an expression which is being evaluated to a single value ([]
, apparently). So what you have is equivalent to:
Oh.
how can i select "embedding" field document whose value is "unset" ?
See https://docs.convex.dev/database/reading-data#combining-operators for how to OR those equality checks
I don't remember, I think
undefined
might be correct (I am not a Convex employee, who would know the answer!)
You could give that a shot!undefined
is correct for finding unset fields. you can do an OR in a filter, but if you want to use the search index to do the filtering (more efficient) you would have to do two fetches and combine them
thx!!
What if I want to do something other than
.eq
with searchIndex "embedding", Actually I wanted something like .notEq
to use with searchIndex "embedding"
for example, "embedding" is notEq
empty array ([]
)
I think I have to use .filter in this case
do you know a better way to do with searchIndex?quick question, are you sure this should be a search index? "userId" sounds like a field that you could check with a regular index
userId is not a document stored in any Table, it is not related to any convex db table.
i get a
userId
from clerkhere's the db schema. there are 2 tables
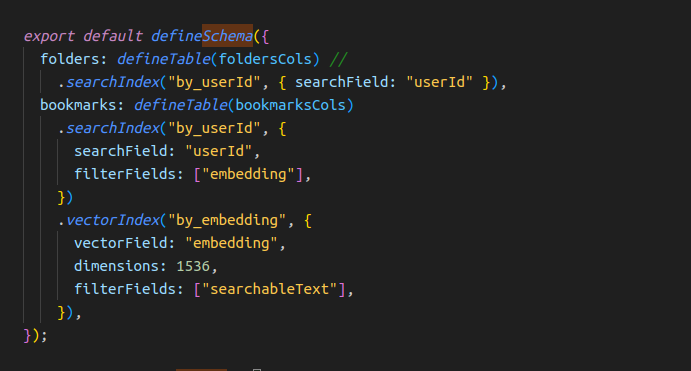
do you want to check that the "userId" field exactly matches
userId
? .searchIndex(...)
does full-text search https://docs.convex.dev/text-search which does not sound like what you want. .index(...)
may be more appropriateFull Text Search | Convex Developer Hub
Run search queries over your Convex documents
Oh understood
thanks!
I liked you searchIndex trick that you showed above.
Do you have any searchIndex trick for this use case? (I want to perform something like
.notEq
)
I think I have to use .filter
if you can use a regular index, you can do a similar trick with
.withIndex("by_userId", q => q.eq("userId", userId).gt("embedding", [])
and then .withIndex("by_userId", q => q.eq("userId", userId).lt("embedding", [])
and then combine them
i brought up the search index thing because search indexes do not support .gt
and .lt
, but regular indexes do. and notEq
is equivalent to a union of gt
and lt
alright! got it
thanks
I'd add that it's likely a single user won't have many associated bookmarks, and so excluding them from the database query won't help much . I would exclude the user after you
.collect()
all your results: