Suspense or loading state
Convex has any loading state or works with suspense or something like?
15 Replies
useQuery
returns undefined
while the data is being loaded.
Check out https://docs.convex.dev/client/react#fetching-dataConvex React | Convex Developer Hub
Convex React is the client library enabling your React application to interact
You could implement a wrapper hook on top
useQuery
that works with Suspense (ref with a promise, resolve it when the data is no longer undefined
). I don't think we have a demo for this yet.interesting...
dredging this up because I was just going to make a suggestion for this! In the meantime I handrolled my own hacky version:
This is great. Thought while reading it:
- queries that return falsey values like
0
or false
or ""
won't work here, should be === undefined
- the order in which watch.onUpdate(cb)
callbacks are called I think is the opposite of what you'd hope here (the resolve()
onUpdate runs before the setState
onUpdate). I don't know suspense well enough to say for sure but I bet that's fine? dunno if resolving the promise synchronously causes React to render
and the main thought while reading it is "we should have a demo of this hook"the order in which watch.onUpdate(cb) callbacks are called I think is the opposite of what you'd hope here (the resolve() onUpdate runs before the setState onUpdate). I don't know suspense well enough to say for sure but I bet that's fine? dunno if resolving the promise synchronously causes React to renderthat might be a part of an issue I was running into, I had to update the useEffect to set the state whenever the watch changes: this fixed state being stale when the query args changes, but it may fix the problem you're talking about too the
new Promise
callback runs synchronously though, so this is basically an onUpdate
subscription immediately after a local query checkYeah would also love to see an official implementation of {isLoading} state or an official suspense solution. Checking if data is undefined is not always enough to cover all UX patterns.
Sometimes you need loading state, empty state and a state with returned items
this is a solution I came up with:
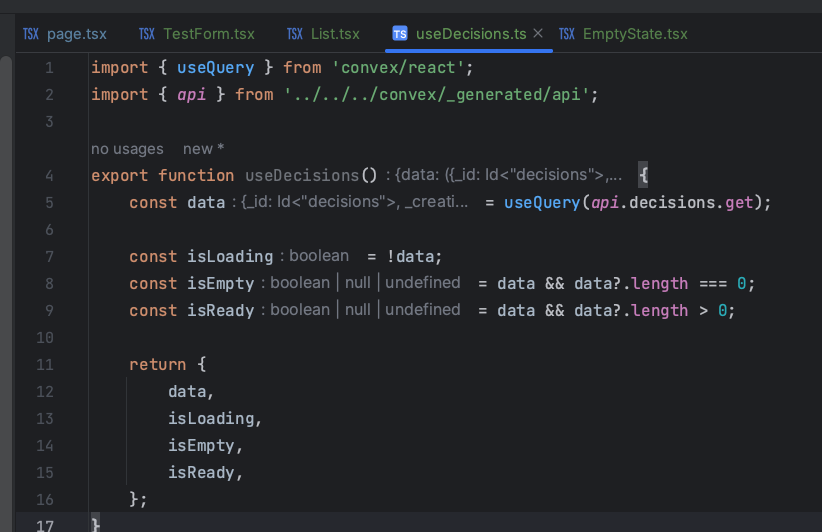
@rutenisraila this is how you can cover all the cases:
īģŋ
We'll work this into the docs, thanks for the feedback!
thanks @Michal Srb !
was thinking about this too and found this thread. i'm finding myself repeating the following pattern quite often
1. check if loading
2. check if null
3. check if user is authorized
4. finally return
i think my implementation could be the easiest. but pls lemme know if i can make this DRYer
Depends on your app! You could perform the
canWrite
check higher up in the tree.
You probably don't need Authenticated
(if it's the one from convex/react
) if you're already checking the auth on the server.oh thanks! interesting. is it possible to do
canWrite
higher up in the tree if i want to check permission per page? here's the snippet i have.
on a side note, can i get the permissions info on the server side? my guess is no đ
server-side as in your convex function? Just call the function api.db.user.getUserPermissions.
Next.js server-side? You can use a convex client from there if you can setAuth server-side (if you have access to the auth token server-side)
yea was referring to next.js server side. ok good idea! lemme poke around. thanks đ