19 Replies
Currently the id is an object, but it will likely be just a string in the future. Are you talking about how to make the string part (like doc._id.id) into an Id object? That is by
new Id(“mytable”, stringId)
You probably shouldn’t cast a regular string to the ID type, because it won’t work as an argument in any of the functions that expect an id.
This is my function, what is the best workaround now?
I tried to call it via
and got a weird error
Expected 1 arguments, but got 2.ts(2554)
This all looks correct. If you move the Id creation to the previous line, is the error still in
new Id(...)
? and you're importing Id from something like import { Id } from "../convex/_generated/dataModel";
?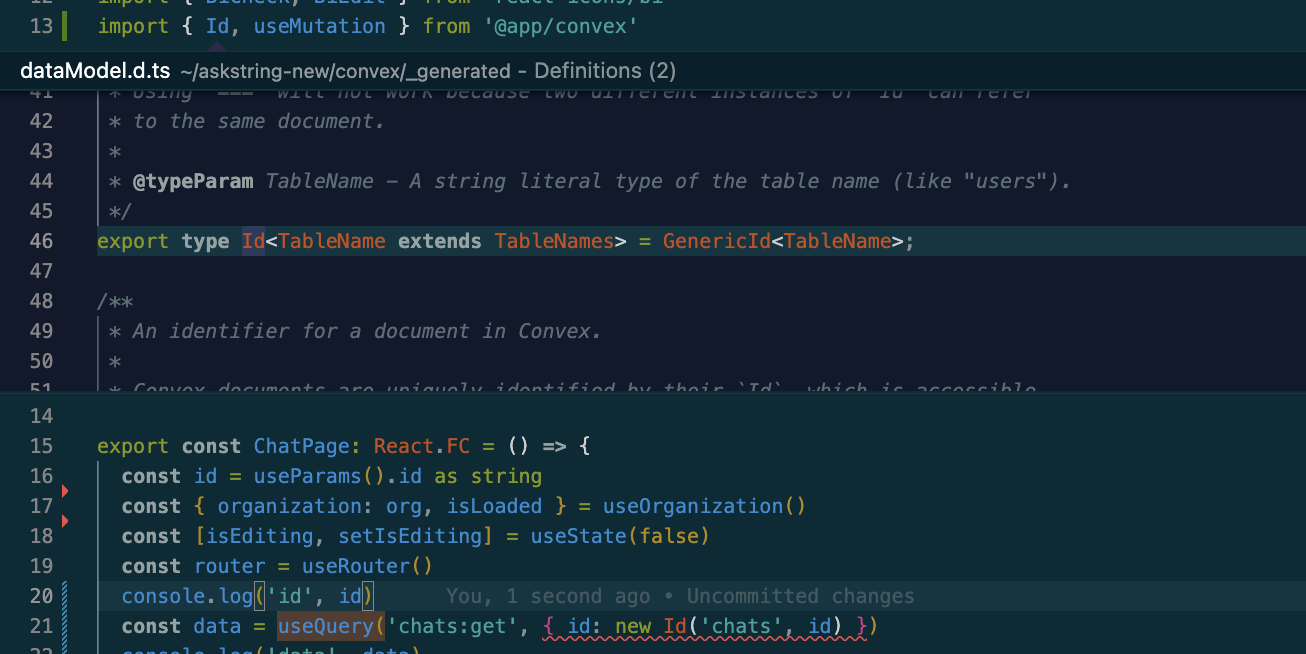
I think so
One thing you'll likely run into: you need to await some of your results. e.g. you need to do
const tools = await Promise.all(chat.tools.map...);
and const messages = await db.query...
and the query needs to call .collect()
oh let me fix them
Does it look correct now? I put await on every line and
collect
in the end
tools will return an array of promises, not a promise itself, so you need to wrap the array of promises with Promise.all() to make it a promise that awaits all the promises in the list
And your console.log shows id as a string and not as undefined? if it's undefined, the Id constructor might think it's only being called with one parameter
yea Id is a string

and what's the type error on the
new Id(...)
? maybe move that to the line above, like const chatId = new Id(...)
to figure out which part is causing the error. I'm assuming you're running convex dev
/ your functions are deployed?yea I am running
npx convex dev
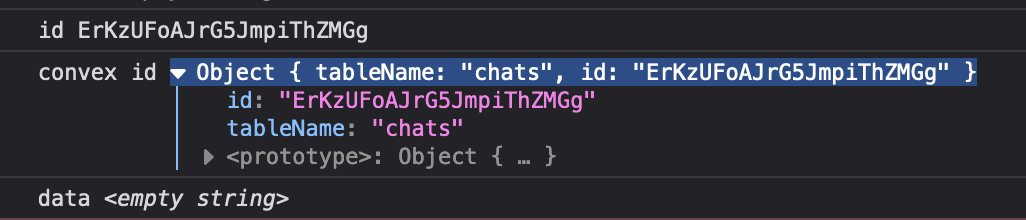
that line looks fine
ah maybe it is my problem having two generated folder
how did you get two? i'm curious
I moved the folders around, but anyways I don't think that's the problem
I've got to run now, but it sounds like the Id generation isn't the issue anymore, so I'll let you figure it out from here. holler if you need more help
I was importing the tanstack
useQuery
😂 , dumb mistake
thanks IanHappens to the best of us!
As an update, IDs are now strings - so
new Id
isn't necessary